Updating Items via Link
Use update mode to add permissions to Items, or to resolve ITEM_LOGIN_REQUIRED status
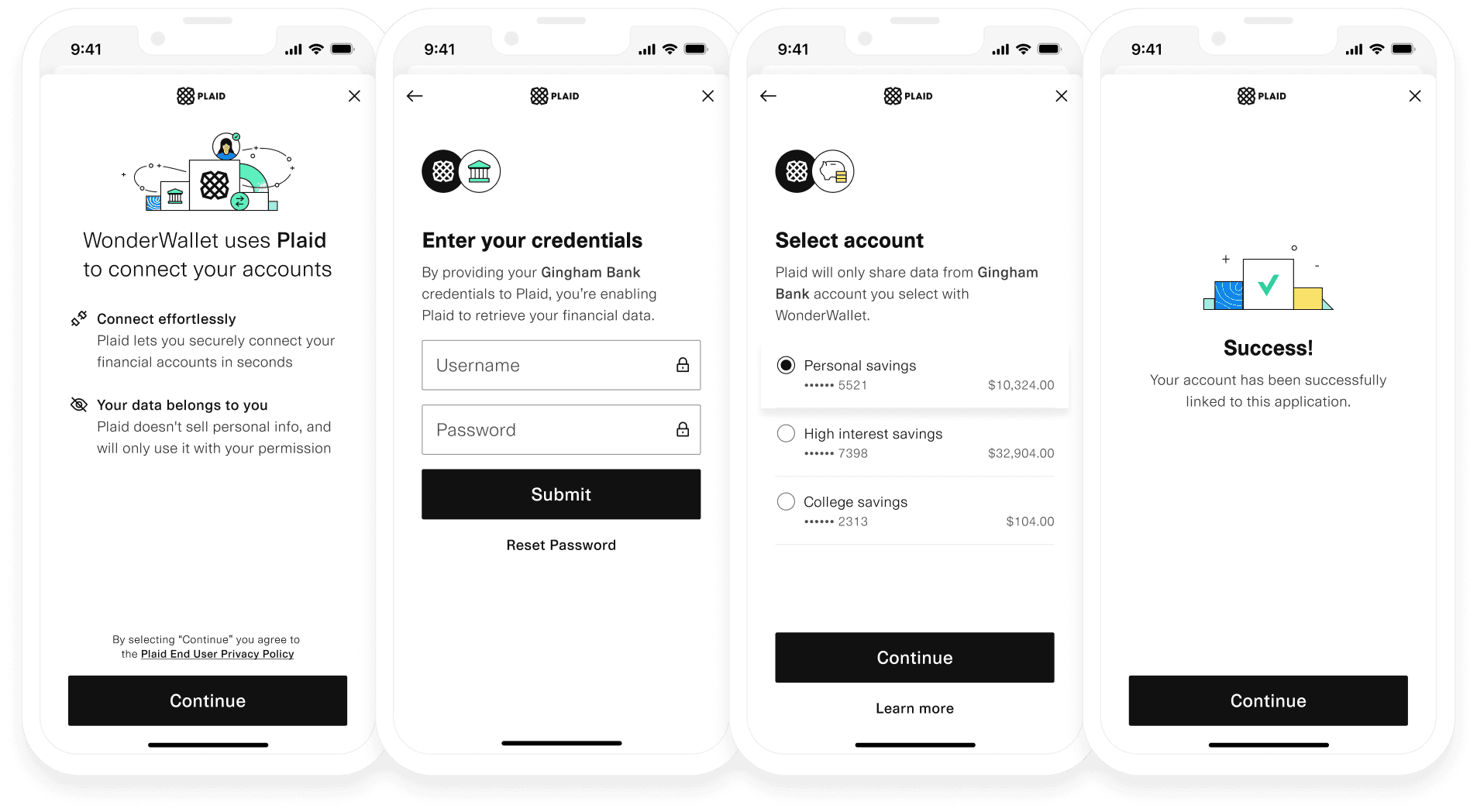
When to use update mode
Update mode refers to any Link session launched for an Item after that Item's initial creation. Update mode is used when an existing Item requires input from a user, such as to update credentials or to grant additional consent.
One common use of update mode is to update authentication or authorization for an Item. This can be required when access to an existing Item stops working: for example, if the end user changes a password on an Item that does not use an OAuth connection, or if authorization for the Item has expired. Update mode can also be used pre-emptively to renew authorization for an Item.
Update mode can also be used to request permissions that the user did not originally grant during the initial Link flow. This can include specific OAuth permissions, access to new accounts, or additional product scopes or use cases. Update mode can be used to manage permissions granted via OAuth, as well as permissions granted directly through Plaid via Account Select or Data Transparency Messaging. When used to request additional permissions, Plaid will show a streamlined Link experience in update mode. For example, if a user is being asked to add a new product, they may not need to log in to their financial institution.
For some older Items created before March 2023, data for all accounts may be available, even if the user did not select the account in Account Select, and access to new accounts may be granted without needing to use the update mode flow. If these Items are at OAuth institutions, they will be updated to use the current Account Select behavior when they are sent through update mode. For more details, see Account Select.
Update mode is also used to launch feature-specific flows that require returning to Link. For example, update mode is used for confirming the micro-deposit verification code in the Same-Day Micro-Deposits Auth flow or to collect statement copies when using Identity Document Upload. For details on using update mode with these flows, see the product-specific pages.
Resolving ITEM_LOGIN_REQUIRED, PENDING_EXPIRATION, or PENDING_DISCONNECT
Receiving an ITEM_LOGIN_REQUIRED
error or a PENDING_EXPIRATION
or PENDING_DISCONNECT
webhook indicates that the Item should be re-initialized via update mode.
If you receive the ITEM_LOGIN_REQUIRED
error after calling a Plaid endpoint, implement Link in update mode during the user flow, and ask the user to re-authenticate before proceeding to the next step in your flow.
If you receive the ITEM_LOGIN_REQUIRED
error via the ITEM: ERROR
webhook, or if you receive either the PENDING_EXPIRATION
or PENDING_DISCONNECT
webhook, re-authenticate with Link in update mode when the user is next in your app. You will need to tell your user (using in-app messaging and/or notifications such as email or text message) to return to your app to fix their Item.
When resolving these issues, for most institutions, Plaid will present an abbreviated re-authentication flow requesting only the minimum user input required to repair the Item. For example, if the Item entered an error state because the user's OTP token expired, the user may be prompted to provide another OTP token, but not to fully re-login to the institution.
Self-healing Item notifications
If a user has connected the same account via Plaid to multiple different apps, resolving the ITEM_LOGIN_REQUIRED
error for an Item in one app may also fix Items in other apps. If one of your Items is fixed in this way, Plaid will notify you via the LOGIN_REPAIRED
webhook. Upon receiving this webhook, you can dismiss any messaging you are presenting to the user telling them to fix their Item via update mode.
Requesting additional products, accounts, permissions, or use cases
You can use update mode to request your users to share new accounts with you. Receiving a NEW_ACCOUNTS_AVAILABLE
webhook indicates that Plaid has detected new accounts that you may want to ask your users to share. For more details, see Using update mode to request new accounts.
Update mode is required when adding Assets, Statements, or Income to an existing Item. For details, see Adding Assets, Statements, or Bank Income.
For Items enabled for Data Transparency Messaging, update mode is required to add additional product and use case consents. For more details, see the Data Transparency Messaging section of this page.
Update mode can optionally be used for resolving errors when adding Auth or Identity; for more details, see Resolving ACCESS_NOT_GRANTED
or NO_AUTH_ACCOUNTS
errors via product validations.
Update mode can be used to request OAuth permissions: triggering update mode for an OAuth institution will cause the user to re-enter the OAuth flow, where they can then grant any required OAuth permissions they failed to grant originally, or restore OAuth permissions they may have revoked. The update mode flow for OAuth institutions will also contain guidance recommending which permissions the user should grant: for more details, see the OAuth documentation.
When an Item is sent through update mode, users can also choose to revoke access they had previously granted. If you lose access to necessary accounts or OAuth permissions after the user completes the update mode flow, you may need to send the user through update mode again. For more details, see Managing consent revocation. To prevent users from revoking access to the Auth or Identity products, you can also use Update Mode with Product Validations.
Renewing consent
Update mode can be used to renew consent on an Item. This requires the user to review their previously shared account and data scopes, and to go through the OAuth flow for applicable institutions. After a user reauthorizes consent, Plaid will update the consent expiration timestamp on the Item to be 12 months in the future.
If you send an Item through update mode and the Item is enabled for Data Transparency Messaging and expiring within the next six months, Plaid will automatically prompt the user to reauthorize consent. To override this default behavior, you can set the update.reauthorization_enabled
field to true
or false
when calling /link/token/create
. Enabling reauthorization on an update mode session may increase the number of steps that the user must take in the Link flow.
For more details on Item expiration, see the Data Transparency Messaging migration guide.
Using update mode
With an access token
To use update mode for an Item, initialize Link with a link_token
configured with the access_token
for the Item that you wish to update.
With a user token
To use update mode for a user, initialize Link with a link_token
configured with the user_token
for the user whose items you wish to update and the update.user
field set to true
. This is the only way to use update mode if you are using Plaid Check products. In Link, the user will be prompted to repair the broken Item. If there are multiple Items in need of repair, the user will be prompted to fix the most recently broken Item. To repair all items associated with a user, you will need to send the user through Link once for each broken Item (you can reuse the same Link token). You can retrieve the status of all Items associated with a user by calling the /user/items/get
endpoint.
With a transfer authorization id
To use update mode for a Transfer authorization with the user_action_required
decision, initialize Link with a link_token
configured with the authorization_id
for the authorization that you wish to update. For more details, see Handling user action required.
Sample code
No products should be added to the products
array and no product-specific request parameters should be specified when creating a link_token
for update mode, unless you are using update mode to add Assets, Statements, or Income, or if you are using Auth and/or Identity and wish to use the beta Update Mode with Product Validations flow. If you are using update mode to add consented products, the products must be added to the additional_consented_products
array instead.
You can obtain a link_token
using the /link/token/create
endpoint.
If your integration uses redirect URIs normally, create the Link token with a redirect URI, as described in Configure your Link token with your redirect URI.
Select group for content switcher1// Create a one-time use link_token for the Item.2// This link_token can be used to initialize Link3// in update mode for the user4const configs = {5 user: {6 client_user_id: 'UNIQUE_USER_ID',7 },8 client_name: 'Your App Name Here',9 country_codes: [CountryCode.Us],10 language: 'en',11 webhook: 'https://webhook.sample.com',12 redirect_uri: "https://www.sample.com/redirect.html",13 access_token: 'ENTER_YOUR_ACCESS_TOKEN_HERE',14};15app.post('/create_link_token', async (request, response, next) => {16 const linkTokenResponse = await client.linkTokenCreate(configs);17
18 // Use the link_token to initialize Link19 response.json({ link_token: linkTokenResponse.data.link_token });20});
Link auto-detects the appropriate institution and handles the credential and multi-factor authentication process, if needed.
An Item's access_token
does not change when using Link in update mode, so there is no need to repeat the exchange token process. Likewise, a User's user_token
does not change when using Link in update mode, so there is no need to create a new user_token
.
1// Initialize Link with the token parameter2// set to the generated link_token for the Item3const linkHandler = Plaid.create({4 token: 'GENERATED_LINK_TOKEN',5 onSuccess: (public_token, metadata) => {6 // You do not need to repeat the /item/public_token/exchange7 // process when a user uses Link in update mode.8 // The Item's access_token has not changed.9 },10 onExit: (err, metadata) => {11 // The user exited the Link flow.12 if (err != null) {13 // The user encountered a Plaid API error prior14 // to exiting.15 }16 // metadata contains the most recent API request ID and the17 // Link session ID. Storing this information is helpful18 // for support.19 },20});
If the Item is enabled for Data Transparency Messaging and consent is expiring within the next six months, Plaid will automatically prompt the user to reauthorize consent during the update mode flow. To override this default behavior, you can set the update.reauthorization_enabled
field to true
or false
when calling /link/token/create
.
When an Item is restored from the ITEM_LOGIN_REQUIRED
state via update mode, if it has been initialized with a product that sends Item webhooks (such as Transactions or Investments), the next webhook fired for the Item will include data for all missed information back to the last time Plaid made a successful connection to the Item.
If the Item has been initialized with Transactions, and was in an error state for over 24 hours, Plaid will check for new transactions immediately after update mode is complete.
Using update mode to request new accounts
The use of update mode to select new accounts is not available for end user financial institution accounts in the UK or EU. In those regions, delete the old Item using /item/remove
and create a new one instead.
You can allow end users to add new accounts to an Item by enabling Account Select when initializing Link in update mode. To do so, first initialize Link for update mode by creating a link_token
using the /link/token/create
endpoint, passing in the access_token
and other parameters as you normally would in update mode.
In addition, make sure you specify the following:
The
update.account_selection_enabled
flag set totrue
.(Optional) A
link_customization_name
. The settings on this customization will determine which View Behavior for the Account Select pane is shown in the update mode flow.(Optional) Any
account_filters
to specify account filtering. Note that this field can only be set for update mode ifupdate.account_selection_enabled
is set totrue
.
Once your user has updated their account selection, all selected accounts will be shared in the accounts
field in the onSuccess()
callback from Link. Any de-selected accounts will no longer be shared with you. You will only be able to receive data for these accounts the user selects in update mode going forward.
Adding an account through update mode will cause Plaid to fetch data for the newly added account. However, recurring transactions streams will not be calculated for the new account until either the next periodic transactions update for the Item occurs or the /transactions/refresh
endpoint is called.
This update mode flow can also be used to remove accounts from an Item. We recommend that you remove any data associated with accounts that your user has de-selected. Note that Chase is an exception to the ability to remove accounts via update mode; to remove access to a specific account on a Chase Item, the end user must do so through the online Chase Security Center.
1curl -X POST https://sandbox.plaid.com/link/token/create \2-H 'Content-Type: application/json' \3-d '{4 "client_id": "${PLAID_CLIENT_ID}",5 "secret": "${PLAID_SECRET}",6 "client_name": "My App",7 "user": { "client_user_id": "${UNIQUE_USER_ID}" },8 "country_codes": ["US"],9 "language": "en",10 "webhook": "https://webhook.sample.com",11 "access_token": "${ACCESS_TOKEN}",12 "link_customization_name": "account_selection_v2_customization",13 "redirect_uri": "https://www.sample.com/redirect.html",14 "update": { "account_selection_enabled": true }15}'
When using the Assets product specifically, if a user selects additional accounts during update mode but does not successfully complete the Link flow, Assets authorization will be revoked from the Item. If this occurs, have the user go through a new Link flow in order to generate an Asset Report, or, if you have Data Transparency Messaging enabled, use update mode to re-authorize the Item for assets, as described in the next section.
If you are using update mode to add a debitable checking or savings account in response to a NO_AUTH_ACCOUNTS
error, see Resolving ACCESS_NOT_GRANTED
or NO_AUTH_ACCOUNTS
errors via product validations for a better method to resolve this error.
Resolving ACCESS_NOT_GRANTED or NO_AUTH_ACCOUNTS errors via Product Validations
Update Mode with Product Validations is currently in beta. To request access, contact support or your Plaid account manager. If you attempt to use this flow before being granted access, the update mode flow will work, but the product validations will not be applied.
The Auth and Identity products can be added to an Item post-Link, by calling an Auth or Identity related endpoint, rather than including auth
or identity
in the products array. However, if the user did not share the necessary permissions or accounts to support these products, the Item will return an ACCESS_NOT_GRANTED
or NO_AUTH_ACCOUNTS
error. Update Mode with Product Validations (UMPV) applies product-specific validations to the selections a user makes in the update mode flow, resulting in higher conversion than resolving these errors via regular update mode.
The process to use UMPV is the same as described in Using update mode, except that you will also include the products
array in the request with the value auth
and/or identity
for the product(s) you wish to validate. You must use the products
array with UMPV; including auth
or identity
in other fields such as required_if_supported_products
or optional_products
will not activate UMPV.
UMPV will enforce the same level of product validation as is normally used on an initial Link attempt: the user will be instructed on which permissions to grant, and if they do not make these selections, they will be prompted to go back through the flow. In the case of NO_AUTH_ACCOUNTS
, the account selection flow will also be automatically enabled if necessary.
UMPV can also be used preventatively, to prevent users in the update mode flow from accidentally removing permissions they have already granted. Applying UMPV to any update mode session for an Auth- or Identity-enabled Item will prompt users to fix their selections if they remove the accounts or permissions required for these products.
UMPV can only be used for auth
or identity
. It is also not compatible with Adding Assets, Statements, or Bank Income; the two cannot be used in a single update mode flow, although they can be used on the same Item, via separate update mode sessions.
Adding Assets, Statements, or Bank Income
Because Assets, Statements, and Income may be used for underwriting use cases, they have unique consent flows in Link. To add one of these products to an Item that did not previously have it enabled, you will need to send the user through update mode so that they can go through the product-specific consent flow before the product is added to the Item.
The process to do this is the same as described in Using update mode, except that you will also include the products
array in the request with the value assets
, statements
, or income_verification
for the product you wish to add.
If the user connected their account less than two years ago, they can bypass the Link credentials pane and complete just the consent panes. Otherwise, they will be prompted to complete the full flow.
Data Transparency Messaging
For Items that are enabled for Data Transparency Messaging (DTM), update mode is required for any of the following: requesting additional consented products, requesting additional consented use cases, or renewing consent.
Requesting additional consented products
For Items with Data Transparency Messaging enabled, you need user consent to access new products. To do so, initialize Link for update mode by creating a link_token
using the /link/token/create
endpoint and passing in the access_token
. For OAuth institutions, ensure you configure the Link token with a redirect URI, as described in Configure your Link token with your redirect URI.
In addition, make sure you specify the following when calling /link/token/create
to add an additional consented product in update mode:
- The
additional_consented_products
field should be set to include any new products you want to gather consent for.- For example, if Link was initialized with just Transactions and you want to upgrade to Identity, you would pass in
identity
to theadditional_consented_products
field. - To see the currently authorized and consented products on an Item, use the
/item/get
endpoint.
- For example, if Link was initialized with just Transactions and you want to upgrade to Identity, you would pass in
- The
link_customization_name
should be set to a customization with Data Transparency Messaging enabled. The use case string should also be broadened to include your reasons for accessing the new data. If the use case is not customized, the default use case will be present on the Data Transparency Messaging pane.
If the upgrade was successful, you will receive the onSuccess()
callback and you will have access to the API endpoints for all of the products you passed into Link update mode. The new products will only be billed once the related API endpoints are called, even if they would otherwise be billed upon Item initialization (e.g., Transactions).
When using update mode to add product consent for an Item with DTM enabled, you must use the additional_consented_products
parameter, not the products
parameter, unless you are adding Assets, Statements, or Income or using Product Validations (beta).
Requesting additional use cases
For Items with DTM enabled, your user will be prompted to consent to their data being used for specific use cases, not just specific products. If you need to obtain consent for additional use cases, you can update your Link customization to add the use cases, then send your user through update mode.
Renewing consent
To renew consent for an Item with DTM enabled, send it through the update mode flow. For details on DTM Item expiration, see Consent expiration and reauthorization.
Testing update mode
Update mode can be tested in the Sandbox using the /sandbox/item/reset_login
endpoint, which will force a given Item into an ITEM_LOGIN_REQUIRED
state.
Example React code in Plaid Pattern
For a real-life example that illustrates the handling of update mode, see linkTokens.js and LaunchLink.tsx. These files contain the Link update mode code for the React-based Plaid Pattern sample app.
Account selection screens in update mode
To demonstrate the user experience of various update mode flows, below are screenshots of update mode that correspond to different Account Select V2 settings.
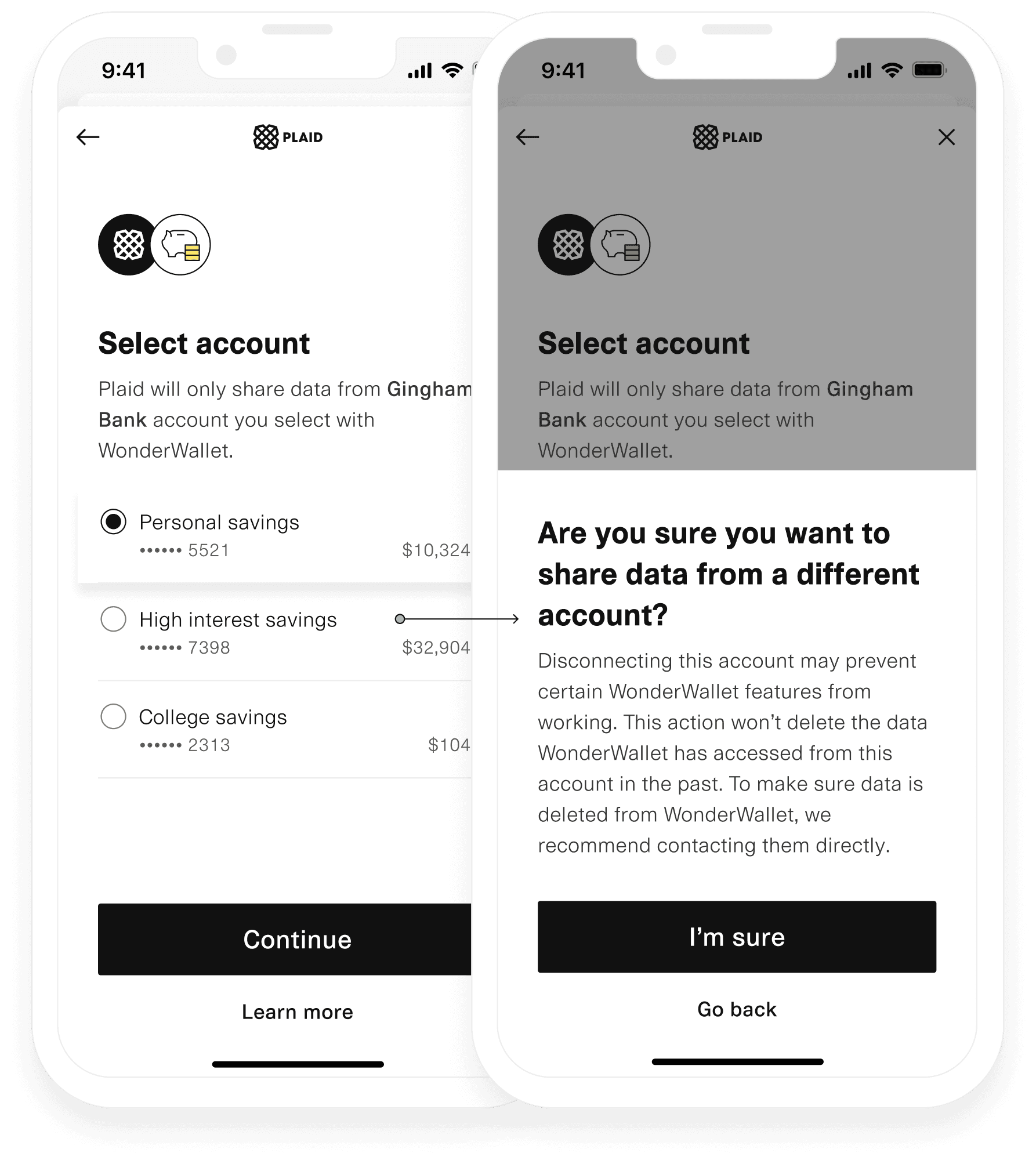
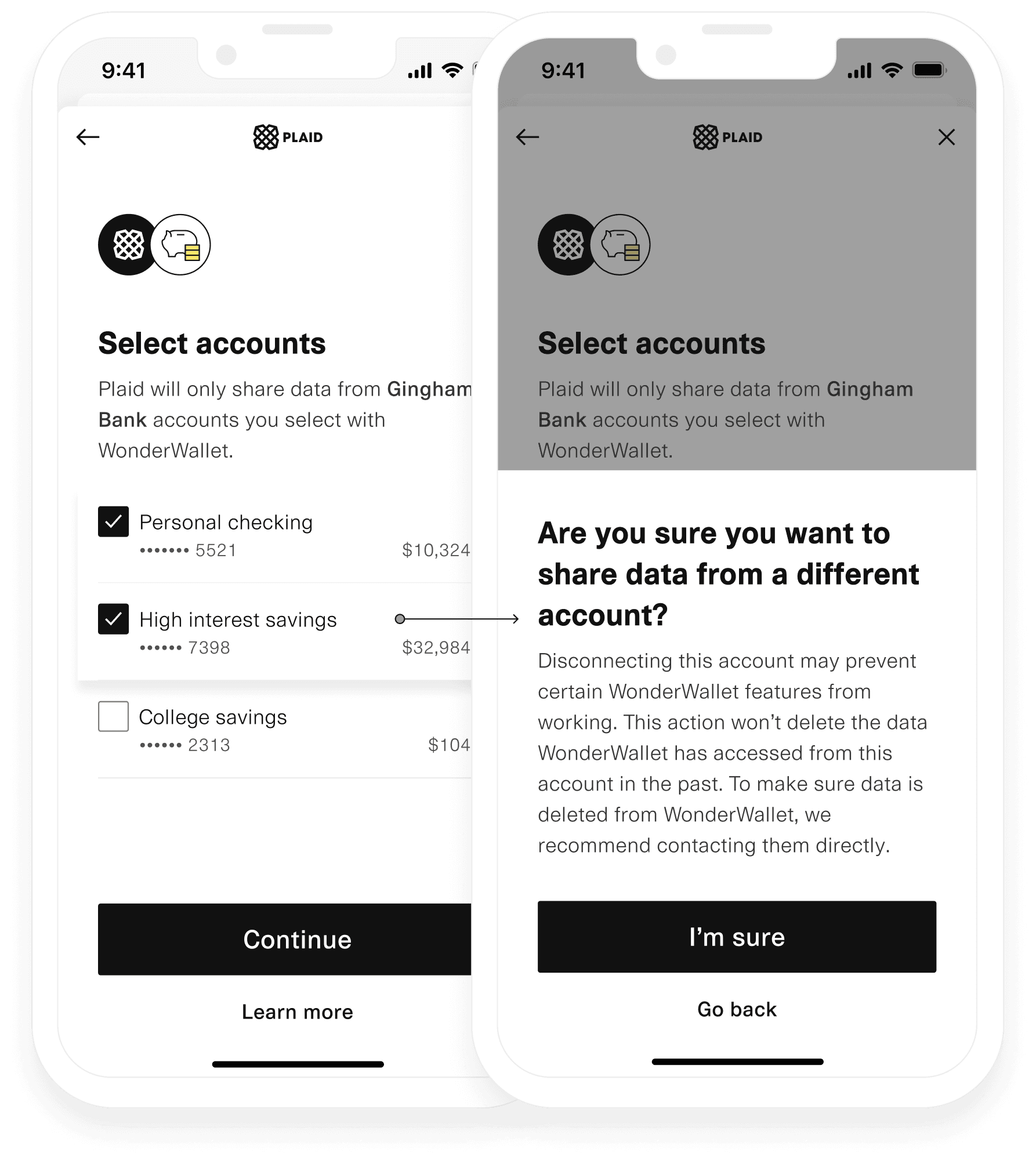
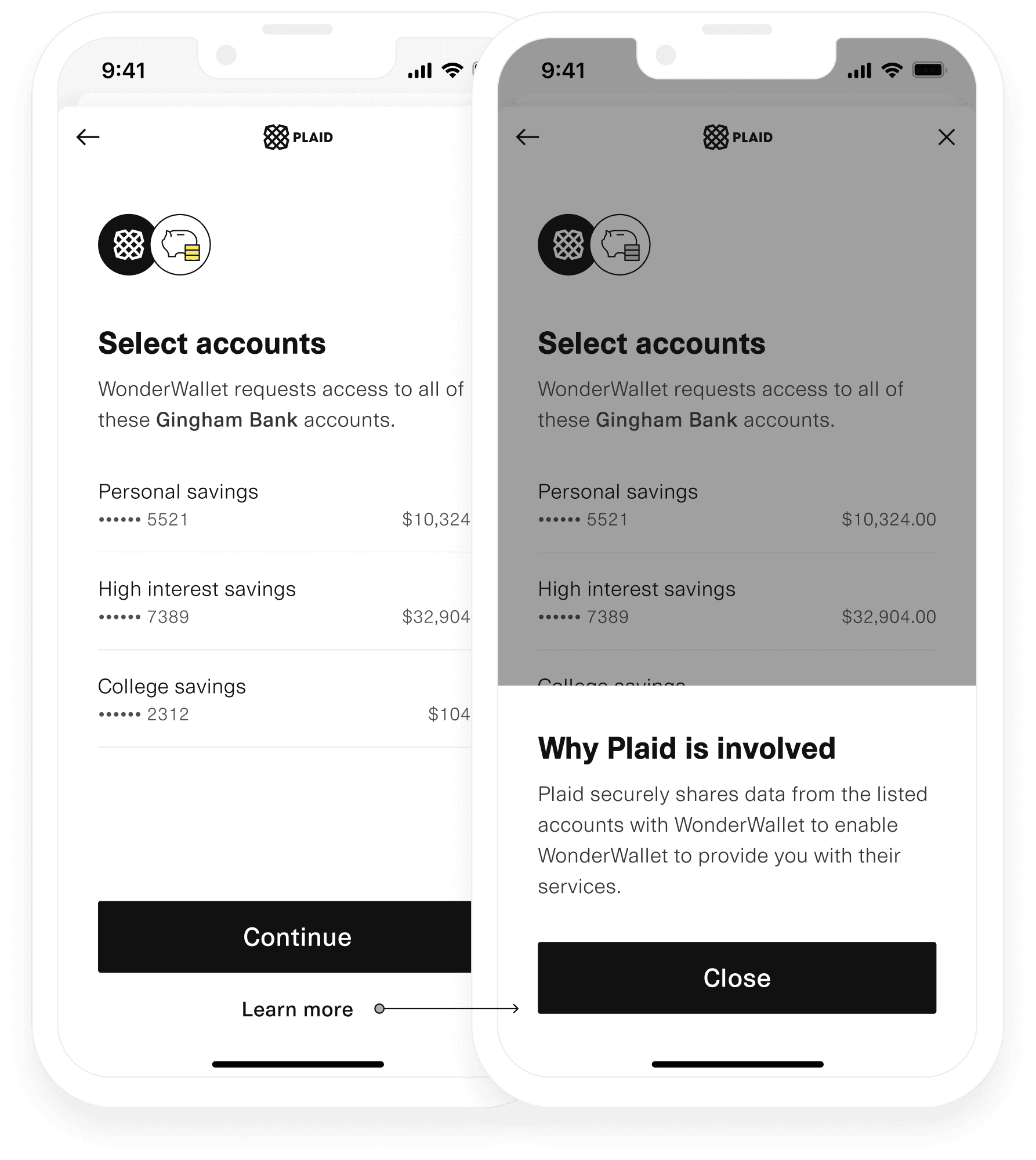