Same Day Micro-deposits
Learn how to authenticate your users with a manually verified micro-deposit
Overview
Same Day Micro-deposits can be used to authenticate any bank account in the US, but especially for the ~2,000 institutions that don't support Instant Auth, Instant Match, or Automated Micro-deposit verification. Plaid will make a deposit that will post within one business day (using Same Day ACH, which is roughly two days faster than the standard micro-deposit experience of two to three days). Users are instructed to manually verify the code in the transaction description deposited in the account.
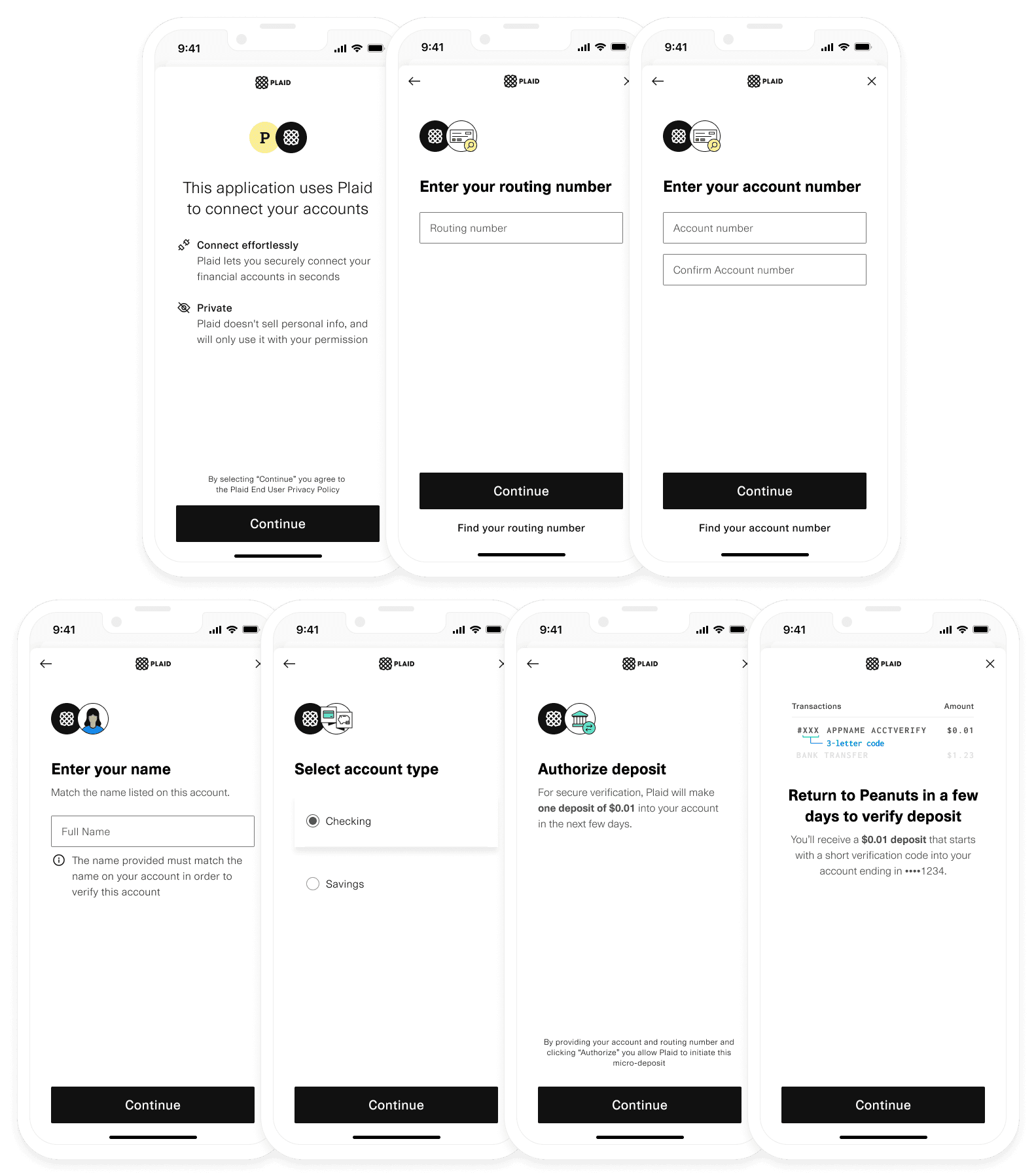
The Same Day Micro-deposit flow
A user connects their financial institution using the following connection flow:
- Starting on a page in your app, the user clicks an action that opens Plaid Link with the correct Auth configuration.
- Inside of Plaid Link, the user enters the micro-deposit initiation flow and provides their legal name, account and routing number.
- Upon successful authentication, Link closes with a
public_token
and ametadata
account status ofpending_manual_verification
. - Behind the scenes, Plaid sends a micro-deposit to the user's account that will post within one to two business days.
- After one to two days, the user is prompted to verify the code in the transaction description in their account, by
opening Link with a generated
link_token
.
Plaid will not reverse the $0.01 micro-deposit credit.
When these steps are done, your user's Auth data is verified and ready to fetch.
Demoing the flow in Link
You can try out the Same Day Micro-deposit flow in Link Demo. For instructions on triggering the flow, see the testing guide.
Implementation steps
Create a link_token
Create a link_token
with the following parameters:
products
array should include onlyauth
ortransfer
as a product when using same-day manual micro-deposit verification. While in most cases additional products can be added to existing Plaid Items, Items created for Same Day manual micro-deposit verification are an exception and cannot be used with any Plaid products other than Auth or Transfer.
Approximately 30% of Items verified by Same Day micro-deposits can also be verified by /identity/match
or /signal/evaluate
. For more details, see Identity Match and Signal.
country_codes
set to['US']
– Micro-deposit verification is currently only available in the United States.auth
object should specify"same_day_microdeposits_enabled": true
1const request: LinkTokenCreateRequest = {2 user: { client_user_id: new Date().getTime().toString() },3 client_name: 'Plaid App',4 products: [Products.Auth],5 country_codes: [CountryCode.Us],6 webhook: 'https://webhook.sample.com',7 language: 'en',8 auth: {9 same_day_microdeposits_enabled: true,10 },11};12try {13 const response = await plaidClient.linkTokenCreate(request);14 const linkToken = response.data.link_token;15} catch (error) {16 // handle error17}
Initialize Link with a link_token
After creating a link_token
for the auth
product, use it to initialize Plaid Link.
When the user successfully inputs their account and routing numbers, the onSuccess()
callback
function will return a public_token
, with verification_status
equal to 'pending_manual_verification'
.
1const linkHandler = Plaid.create({2 // Fetch a link_token configured for 'auth' from your app server3 token: (await $.post('/create_link_token')).link_token,4 onSuccess: (public_token, metadata) => {5 // Send the public_token and connected accounts to your app server6 $.post('/exchange_public_token', {7 publicToken: public_token,8 accounts: metadata.accounts,9 });1011 metadata = {12 ...,13 link_session_id: String,14 institution: {15 name: null, // name is always null for Same Day Micro-deposits16 institution_id: null // institution_id is always null for Same Day Micro-deposits17 },18 accounts: [{19 id: 'vzeNDwK7KQIm4yEog683uElbp9GRLEFXGK98D',20 mask: '1234',21 name: "Checking...1234" | "Savings...1234",22 type: 'depository',23 subtype: 'checking' | 'savings',24 verification_status: 'pending_manual_verification'25 }]26 }27 },28 // ...29});3031// Open Link on user-action32linkHandler.open();
Display a "pending" status in your app
Because Same Day verification usually takes one business day to complete, we recommend displaying a UI in your app that communicates to a user that verification is currently pending.
You can use the verification_status
key returned in the onSuccess
metadata.accounts
object once
Plaid Link closes successfully.
1verification_status: 'pending_manual_verification';
You can also fetch the verification_status
for an
Item's account via the Plaid API, to obtain the latest account status.
Exchange the public token
In your own backend server, call the /item/public_token/exchange
endpoint with the Link public_token
received in the onSuccess
callback to
obtain an access_token
. Persist the returned access_token
and item_id
in your database
in relation to the user.
When using same-day micro-deposit verification, only one account can be associated with each access token. If you want to allow a user to link multiple accounts at the same institution using same-day micro-deposits, you will need to create a new Link flow and generate a separate access token for each account.
To test your integration outside of Production, see Testing Same Day Micro-deposits in Sandbox.
Select group for content switcher1// publicToken and accountID are sent from your app to your backend-server2const accountID = 'vzeNDwK7KQIm4yEog683uElbp9GRLEFXGK98D';3const publicToken = 'public-sandbox-b0e2c4ee-a763-4df5-bfe9-46a46bce993d';45// Obtain an access_token from the Link public_token6const response = await client7 .itemPublicTokenExchange({8 public_token: publicToken,9 })10 .catch((err) => {11 // handle error12 });13const accessToken = response.access_token;
1{2 "access_token": "access-sandbox-5cd6e1b1-1b5b-459d-9284-366e2da89755",3 "item_id": "M5eVJqLnv3tbzdngLDp9FL5OlDNxlNhlE55op",4 "request_id": "m8MDnv9okwxFNBV"5}
Check the account verification status (optional)
In some cases you may want to implement logic in your app to display the verification_status
of
an Item that is pending manual verification. The /accounts/get
API endpoint allows you to query this information.
To be notified via webhook when Plaid has sent the micro-deposit to your end user, see micro-deposit events.
1// Fetch the accountID and accessToken from your database2const accountID = 'vzeNDwK7KQIm4yEog683uElbp9GRLEFXGK98D';3const accessToken = 'access-sandbox-5cd6e1b1-1b5b-459d-9284-366e2da89755';4const request: AccountsGetRequest = {5 access_token: accessToken,6};7const response = await client.accountsGet(request).catch((err) => {8 // handle error9});10const account = response.accounts.find((a) => a.account_id === accountID);11const verificationStatus = account.verification_status;
1{2 "accounts": [3 {4 "account_id": "vzeNDwK7KQIm4yEog683uElbp9GRLEFXGK98D",5 "balances": { Object },6 "mask": "0000",7 "name": "Checking...0000",8 "official_name": null,9 "type": "depository"10 "subtype": "checking" | "savings",11 "verification_status":12 "pending_manual_verification" |13 "manually_verified" |14 "verification_failed",15 },16 ...17 ],18 "item": { Object },19 "request_id": String20}
Prompt user to verify micro-deposit code in Link
After one to two business days, the micro-deposit sent to the user's account is expected to be posted. To securely verify a Same Day Micro-deposits account, your user needs to come back into Link to verify the code in the transaction description.
When the micro-deposit posts to your end user's bank account, the transaction description will be written with the format:
1#XXX <clientName> ACCTVERIFY
The #
will be followed with the three letter code required for verification. The <clientName>
is defined by the value of
the client_name
parameter that was used to create the link_token
that initialized Link.
Users with business or corporate accounts that have ACH debit blocks enabled on
their account may need to authorize Plaid's Company / Tax ID, 1460820571
, to
avoid any issues with linking their accounts.
To optimize conversion, we strongly recommend sending your user a notification (e.g. email, SMS, push notification) prompting them to come back into your app and verify the micro-deposit code. To be notified via webhook when Plaid has sent the micro-deposit to your end user, see micro-deposit events.
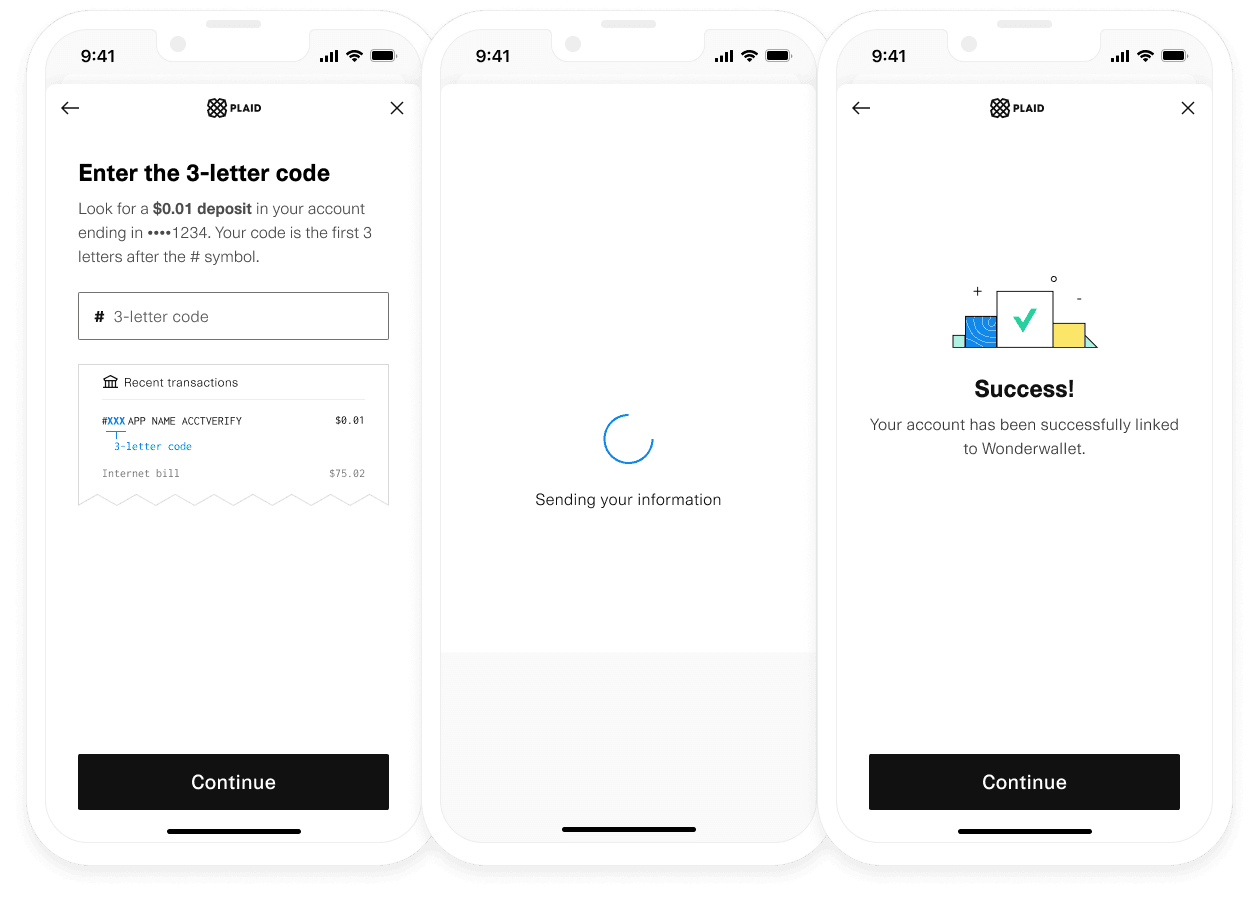
Verification of Same Day Micro-deposits is performed in two steps:
- In your backend server, create a new
link_token
from the associatedaccess_token
for the given user. - Pass the generated
link_token
into your client-side app, using thetoken
parameter in the Link configuration. This will automatically trigger the micro-deposit verification flow in Link.
Create a new link_token from a persistent access_token
Generate a link_token
for verifying micro-deposits by passing the user's associated access_token
to the
/link/token/create
API endpoint. Note that the products
field should not be set because the micro-deposits verification flow does not change the products associated with the given access_token
.
1// Using Express2app.post('/api/create_link_token', async function (request, response) {3 // Get the client_user_id by searching for the current user4 const user = await User.find(...);5 const clientUserId = user.id;6 const request = {7 user: {8 client_user_id: clientUserId,9 },10 client_name: 'Plaid Test App',11 language: 'en',12 webhook: 'https://webhook.sample.com',13 country_codes: [CountryCode.Us],14 access_token: 'ENTER_YOUR_ACCESS_TOKEN',15 };16 try {17 const createTokenResponse = await client.linkTokenCreate(request);18 response.json(createTokenResponse.data);19 } catch (error) {20 // handle error21 }22});
Initialize Link with the generated link_token
In your client-side app, pass the generated link_token
into the Link token
parameter. Link will
automatically detect that Same Day verification is required for the Item and will open directly into
the verification flow (see the image above).
In Link, the user will be prompted to log in to their personal banking portal to confirm the code in the
micro-deposit transaction description. Upon successful entry of the code, the onSuccess
callback will be fired, with an
updated verification_status: 'manually_verified'
. The verification code will be case-insensitive.
There is no time limit for the user to verify the deposit. A user has three attempts to enter the code correctly, after which the Item will be permanently locked for security reasons. See INCORRECT_DEPOSIT_VERIFICATION and PRODUCT_NOT_READY for errors that may occur during the micro-deposit initiation and verification flow.
1const linkHandler = Plaid.create({2 token: await fetchLinkTokenForMicrodepositsVerification(),3 onSuccess: (public_token, metadata) => {4 metadata = {5 accounts: [{6 ...,7 verification_status: 'manually_verified',8 }],9 };10 },11 // ...12});1314// Open Link to verify micro-deposit amounts15linkHandler.open();
An Item's access_token
does not change when verifying micro-deposits, so there is no need to repeat
the exchange token process.
Fetch Auth data
Finally, we can retrieve Auth data once the user has manually verified their account through Same Day Micro-deposits:
Select group for content switcher1const accessToken = 'access-sandbox-5cd6e1b1-1b5b-459d-9284-366e2da89755';23// Instantly fetch Auth numbers4const request: AuthGetRequest = {5 access_token: accessToken,6};7const response = await client.authGet(request).catch((err) => {8 // handle error9});10const numbers = response.numbers;
1{2 "numbers": {3 "ach": [4 {5 "account_id": "vzeNDwK7KQIm4yEog683uElbp9GRLEFXGK98D",6 "account": "1111222233330000",7 "routing": "011401533",8 "wire_routing": "021000021"9 }10 ],11 "eft": [],12 "international": [],13 "bacs": []14 },15 "accounts": [16 {17 "account_id": "vzeNDwK7KQIm4yEog683uElbp9GRLEFXGK98D",18 "balances": { Object },19 "mask": "0000",20 "name": "Checking ...0000",21 "official_name": null,22 "verification_status": "manually_verified",23 "subtype": "checking",24 "type": "depository"25 }26 ],27 "item": { Object },28 "request_id": "m8MDnv9okwxFNBV"29}
Check out the /auth/get
API reference documentation to see the full
Auth request and response schema.
Using Text Message Verification
Text Message Verification is an alternative verification method for the Same Day Micro-deposit flow. With Text Message Verification, Plaid will send your user a one-time SMS message, directing them to a Plaid-hosted website where they can complete the micro-deposit verification process. When the user is done verifying their micro-deposit code, you will receive a SMS_MICRODEPOSITS_VERIFICATION
webhook, telling you that the user has completed the process and that it is now safe to retrieve Auth information.
Text Message Verification can and should be used alongside the usual verification flow of prompting your user to verify their code inside your app through Link. The user may choose not to receive an SMS message from Plaid, or they might simply ignore the message, so it is important for your app to still provide a way for your user to complete the process.
Implementation steps
Text Message Verification is enabled by default as long as Same Day Micro-deposits have been enabled. To opt out of Text Message Verification, add the sms_microdeposits_verification_enabled
boolean to the auth
object in your /link/token/create
configuration, and set it to false
.
When calling
/link/token/create
, make sure you have specified a URL for your webhook receiver, so you can receive theSMS_MICRODEPOSITS_VERIFICATION
webhook.Listen for the
SMS_MICRODEPOSITS_VERIFICATION
webhook.When the user completes the verification process, Plaid will send a
SMS_MICRODEPOSITS_VERIFICATION
webhook to the webhook receiver URL that you specified earlier. When you receive this webhook, review the value of thestatus
field.1{2 "webhook_type": "AUTH",3 "webhook_code": "SMS_MICRODEPOSITS_VERIFICATION",4 "status": "MANUALLY_VERIFIED",5 "item_id": "eVBnVMp7zdTJLkRNr33Rs6zr7KNJqBFL9DrE6",6 "account_id": "dVzbVMLjrxTnLjX4G66XUp5GLklm4oiZy88yK",7 "environment": "sandbox"8}A value of
MANUALLY_VERIFIED
indicates that the user successfully entered the micro-deposit code and has verified their account information. You can now retrieve Auth information on behalf of this user, and you should remove any pending in-app messages asking the user to complete the verification process.If you re-open Link and ask the user to verify their code after they have already verified it using Text Message Verification, Link will close immediately and fire the
onSuccess
callback. So even if you don't act on this webhook, your application will continue to function normally.A
status
field ofVERIFICATION_FAILED
indicates that the user failed the verification process. Verification cannot be retried once this status has been triggered; you will need to create a new Item.
User experience
When the user goes through the Same Day Micro-deposit flow in Link, they will be prompted to enter their phone number. After the micro-deposit has been placed in their account, Plaid will contact the user via SMS with a URL pointing to a Plaid-hosted page where the user can complete the verification process. The text message itself will contain the following message:
1Plaid: On behalf of [client_name], a $0.01 deposit was sent to your account ending in 1234. Verify this deposit here: https://hosted.plaid.com/link/lp1234. Then, return to [client_name] to complete your account setup.
Currently, the text message is only provided in English and will not be localized according to your Link customization settings.
Testing text message verification
Text message verification cannot be tested in the Sandbox environment. Text messages will only be sent in Production.
Same Day Micro-deposit flow configuration options
In addition to the default flow, Same Day Micro-deposits has several optional flow settings you can enable.
To expose more users to the Same Day micro-deposit flow, you can enable Auth Type Select, or to limit users' exposure to the flow, you can enable Reroute to Credentials.
To provide an alternative flow that allows users to skip micro-deposit verification and instead relies on recognizing a known bank account within the Plaid network, you can enable Database Match.
The setting that is best for you will depend on your use case, your risk exposure, and which other Plaid products you use. Learn more about how to optimize your configuration and manage risk under best practices.
Same Day Micro-deposit flow options are configured via the auth
object in the /link/token/create
request. This enables you to decide which sessions are enabled for which flows; for example, you can enable different flows based on users' risk profiles.
Default manual verification flow
For many customers, Same Day Micro-deposits and other manual verification options, such as Database Insights (beta), are best configured as an option when credential-based Auth types fail. This maximizes the conversion of Items that have broader coverage of additional data products (Balance, Identity, Transactions, etc.), but also allows extended Auth coverage. When using the default flow, the Link with account numbers entry point will appear for Same Day Micro-deposits when the user does any of the following:
- Encounters an error in the Link flow
- Selects an institution whose connection health is poor
- Attempts to close the Link modal without connecting an institution
- Receives a "no results" message when searching for an institution
- Scrolls to the bottom of the institution search results
This is the default flow for accessing Same-day micro-deposits and no special configuration settings are required to trigger it.
To trigger this flow via the Link demo specify Auth in the Product dropdown menu and then Launch Demo. Trigger empty search results (type in a search query like ‘xyz’) or the Exit Pane (by closing Link) and select Link with account numbers.
After the user has entered their account and routing number, if the account information matches an institution that supports Instant Micro-deposits, they will automatically be rerouted from the Same Day Micro-deposit flow to the higher-converting Instant Micro-deposit flow.
Database Match
Database Match enables instant manual account verification without the need for micro-deposits, instead relying on Plaid's database of known account numbers. When provided as an alternative to Same-Day Micro-deposits, Database Match can increase conversion, as the user may be able to verify instantly, without having to return to Plaid to verify their micro-deposit codes.
Database Match will present the user with the option to manually add a bank account in Link by providing their name, account number, and routing number. Plaid will then check this information against its network of over 200 million known bank accounts. Approximately 30% of user bank accounts can be verified via Database Match. If a match is not found, Database Match will fall back to routing the user to a manual micro-deposit flow, either Instant Micro-deposits or Same-Day Micro-deposits.
Database Match can be used to verify the validity of the bank account being linked, but it does not verify that the end user has access to the bank account. To mitigate account takeover risk with Database Match, it can be paired with Identity Match to verify the user's information, such as phone number and address, on the linked account. All accounts that can be verified with Database Match can also be used with Identity Match.
Database Match flow
- Starting on a page in your app, the user clicks an action that opens Plaid Link.
- Inside of Plaid Link, the user selects an option to enter the manual verification flow and provides their legal name, account and routing number.
- Plaid will confirm the account number, routing number, and name match a previously verified account.
- If a match is confirmed, Link closes with a
public_token
and a verification status ofdatabase_matched
. - If there is no match, the user will be prompted to enter the Instant or Same Day Micro-deposits flow.
When these steps are complete, you can call /item/public_token/exchange
to obtain an access_token
for calling endpoints such as /auth/get
or /processor/token/create
.
Database Match implementation steps
To enable Database Match, use the same settings as Same-Day Micro-deposits, and add the following parameter when calling /link/token/create
:
auth
object should specify"database_match_enabled": true
.
Database Match cannot be used in the same session as Database Insights. If Database Insights is explicitly enabled in the auth
object alongside Database Match, an error will occur when calling /link/token/create
.
1const request: LinkTokenCreateRequest = {2 user: { client_user_id: new Date().getTime().toString() },3 client_name: 'Plaid App',4 products: [Products.Auth],5 country_codes: [CountryCode.Us],6 webhook: 'https://webhook.sample.com',7 language: 'en',8 auth: {9 database_match_enabled: true,10 same_day_microdeposits_enabled, true11 },12};13try {14 const response = await plaidClient.linkTokenCreate(request);15 const linkToken = response.data.link_token;16} catch (error) {17 // handle error18}
When calling /auth/get
, the returned object will have a verification_status
value of database_matched
as an indication that the user's data was verified through Database Match.
1{2 "numbers": {3 "ach": [4 {5 "account": "1111222233330000",6 "routing": "011401533",7 "wire_routing": "021000021"8 }9 ],10 ...11 },12 "accounts": [13 {14 "account_id": "vzeNDwK7KQIm4yEog683uElbp9GRLEFXGK98D",15 "balances": { Object },16 "mask": "0000",17 "name": "Checking...0000",18 "official_name": null,19 "verification_status": "database_matched",20 "subtype": "checking",21 "type": "depository"22 }23 ],24 ...25}
Testing Database Match in Sandbox
For test credentials that can be used to test Database Match in the Sandbox environment, see Testing Database Match.
Reroute to Credentials
By default, Optional Reroute to Credentials is enabled for all eligible flows. This setting can be configured on a per-session basis via the auth
object in the /link/token/create
request, or you can reach out to your Plaid account manager to change the default setting for all Link flows.
In some cases, when rerouting a user to a supported institution, Plaid may show a list of institutions instead of a single institution. This can occur when a financial institution has multiple different brands that share a common routing number.
Optional Reroute
Optional Reroute to Credentials is the default flow. It is best for customers who would like to make a recommendation for the user to connect via credentials, but not block the user from proceeding
with the Same Day Micro-deposits flow. This flow will provide a reminder to the user that the institution is supported, and detail the benefits of instant connection, while
also leaving the manual verification option in place. To enable Optional Reroute, you do not need to take any action. If you have asked your Account Manager to change your default flow to something else, you can set auth.reroute_to_credentials
to OPTIONAL
in the /link/token/create
call. To disable Optional Reroute, set this value to OFF
.
Single Institution Optional Reroute | Multiple Institution Optional Reroute |
---|---|
![]() | ![]() |
Forced Reroute
Forced Reroute to Credentials is best for customers who would like to restrict the user to connect via credentials if the institution is supported on a credential-based flow
and stop the user from proceeding with the Same Day Micro-deposits flow. This flow is designed to block a user from connecting manually for institutions that are supported
via credentials in high ACH risk use cases. To enable Forced Reroute, set auth.reroute_to_credentials
to FORCED
in the /link/token/create
call.
Single Institution Forced Reroute | Multiple Institution Forced Reroute |
---|---|
![]() | ![]() |
Sample code for reroute to credentials
1const request: LinkTokenCreateRequest = {2 user: { client_user_id: new Date().getTime().toString() },3 client_name: 'Plaid App',4 products: [Products.Auth],5 country_codes: [CountryCode.Us],6 language: 'en',7 auth: {8 same_day_microdeposits_enabled: true,9 reroute_to_credentials: 'OPTIONAL',10 },11};12try {13 const response = await plaidClient.linkTokenCreate(request);14 const linkToken = response.data.link_token;15} catch (error) {16 // handle error17}
Auth Type Select
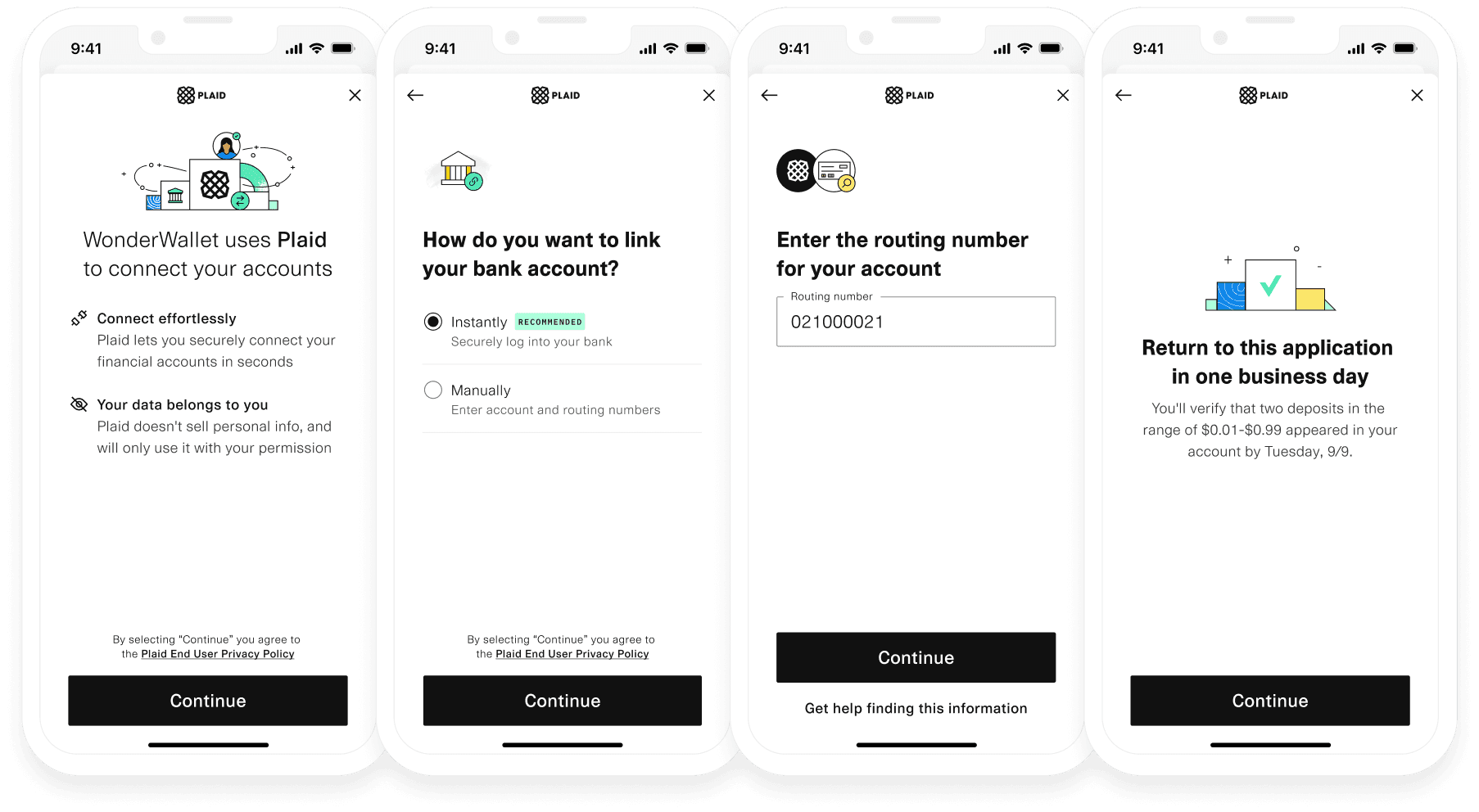
Auth Type Select is a Link configuration of Same Day Micro-deposits which shows a pane upfront, enabling end users to actively choose between instant, credential-based authentication (i.e. Instant Auth, Instant Match, Automated Micro-deposits) and manual account authentication (i.e. Same Day Micro-deposits) at the start of the Plaid Link flow. By offering this choice, you can proactively offer the authentication method for your users who either cannot or do not opt for credential-based Auth Types.
To demo Auth Type Select via the Plaid Link demo, specify United States and Auth in the Country and Product dropdown menu respectively. Toggle on Auth Type Select, then Launch Demo.
To enable Auth Type Select, when calling /link/token/create
, the auth_type_select_enabled
boolean in the auth
object should be set to true
.
When to use Auth Type Select
Auth Type Select is best suited for customers who expect that a substantial portion of their consumers would prefer to authenticate manually, and who prefer to maximize Auth coverage over other data products. Examples of this may include Business Account Verification use cases where a high percentage of users are not expected to have access to credentials of a bank account, yet do have access to the transaction records, and therefore can verify micro-deposits.
Customers that see the greatest success with the Auth Type Select configuration have a substantial portion of users (over half, as a rule of thumb) who cannot or will not connect by logging into their bank, who have invested in fraud risk mitigations or have a low risk use case, and/or have very high intent users (such as users connecting a bank account to receive a payment).
Some customers observe an increase in conversion of verified Auth data with this configuration. However, this configuration reduces coverage of other products (like Balance or Identity) as those products are only available via credential-based Auth types. When offered the manual option upfront, more users may choose to link manually. Users who opt to connect via micro-deposits can have lower conversion than Instant Auth because the flow requires more steps for a user, including returning to Link the next day to verify micro-deposits. It may encourage users to connect via micro-deposits who would otherwise connect via credential-based Auth types (if the micro-deposits option was not available upfront).
This configuration is best for customers who want to maximize Auth coverage and can tolerate reduced coverage of other products like Balance and Identity.
Sample code for Auth Type Select
1const request: LinkTokenCreateRequest = {2 user: { client_user_id: new Date().getTime().toString() },3 client_name: 'Plaid App',4 products: [Products.Auth],5 country_codes: [CountryCode.Us],6 language: 'en',7 auth: {8 same_day_microdeposits_enabled: true,9 auth_type_select_enabled: true,10 },11};12try {13 const response = await plaidClient.linkTokenCreate(request);14 const linkToken = response.data.link_token;15} catch (error) {16 // handle error17}
Handling Link events
When a user goes through the Same Day micro-deposits flow, the session will have the TRANSITION_VIEW (view_name = NUMBERS)
event, and in the onSuccess
callback the verification_status
will be pending_manual_verification
because the user will have to return to Link to verify their micro-deposit at a later Link session.
1OPEN (view_name = CONSENT)2TRANSITION_VIEW (view_name = SELECT_INSTITUTION)3SEARCH_INSTITUTION4TRANSITION_VIEW (view_name = NUMBERS)5TRANSITION_VIEW (view_name = LOADING)6TRANSITION_VIEW (view_name = CONNECTED)7HANDOFF8onSuccess (verification_status: pending_manual_verification)
When a user goes through the Same Day micro-deposits flow with Reroute to Credentials, you will additionally see TRANSITION_VIEW (view_name = NUMBERS_SELECT_INSTITUTION)
with view_variant = SINGLE_INSTITUTION
or view_variant = MULTI_INSTITUTION
.
1OPEN (view_name = CONSENT)2TRANSITION_VIEW (view_name = SELECT_INSTITUTION)3SEARCH_INSTITUTION4TRANSITION_VIEW (view_name = NUMBERS)5TRANSITION_VIEW (view_name = NUMBERS_SELECT_INSTITUTION, view_variant = SINGLE_INSTITUTION)6TRANSITION_VIEW (view_name = LOADING)7TRANSITION_VIEW (view_name = CONNECTED)8HANDOFF9onSuccess (verification_status: pending_manual_verification)
When a user goes through the Same Day micro-deposits flow with the Auth Type Select configuration, you will additionally see TRANSITION_VIEW (view_name = SELECT_AUTH_TYPE)
and also SELECT_AUTH_TYPE (selection = flow_type_manual)
1OPEN (view_name = CONSENT)2TRANSITION_VIEW (view_name = SELECT_AUTH_TYPE)3SELECT_AUTH_TYPE (selection = flow_type_manual)4TRANSITION_VIEW (view_name = NUMBERS)5TRANSITION_VIEW (view_name = LOADING)6TRANSITION_VIEW (view_name = CONNECTED)7HANDOFF8onSuccess (verification_status: pending_manual_verification)