Link Android SDK
Learn how to integrate your app with the Link Android SDK
Overview
The Plaid Link SDK is a quick and secure way to link bank accounts to Plaid in your Android app. Link is a drop-in module that handles connecting a financial institution to your app (credential validation, multi-factor authentication, error handling, etc), without passing sensitive personal information to your server.
To get started with Plaid Link for Android, clone the GitHub repository and try out the example application, which provides a reference implementation in both Java and Kotlin. Youʼll want to sign up for free API keys through the Plaid Dashboard to get started.
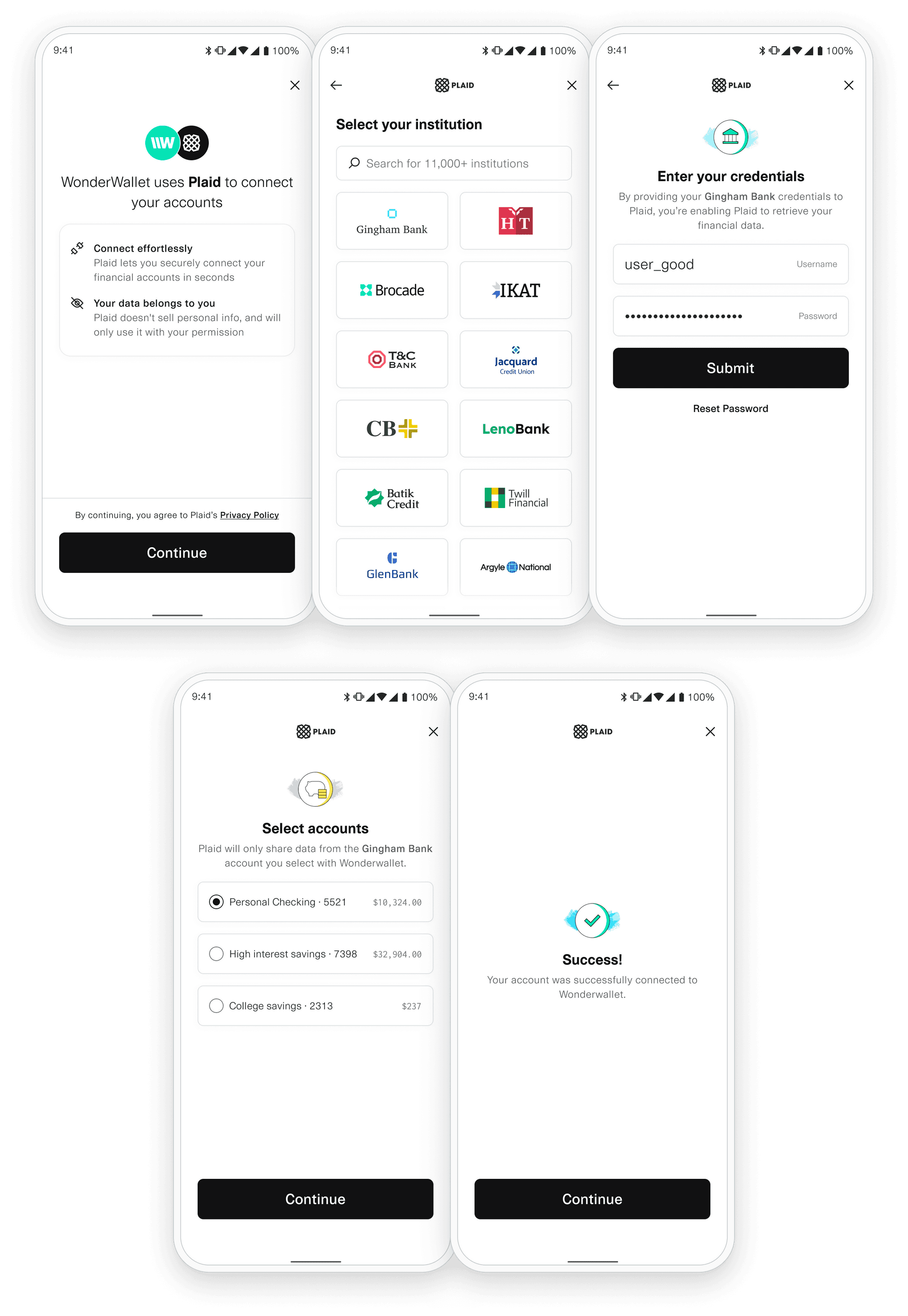
Prefer to learn by watching? A video guide is available for this content.
Initial Android setup
Before writing code using the SDK, you must first perform some setup steps to register your app with Plaid and configure your project.
Register your app ID
To register your Android app ID:
- Sign in to the Plaid Dashboard and go to the Developers -> API page.
- Next to Allowed Android Package Names click Configure then Add New Android Package Name.
- Enter your package name, for example
com.plaid.example
. - Click Save Changes.
Your Android app is now set up and ready to start integrating with the Plaid SDK.
New versions of the Android SDK are released frequently, at least once every few months. Major releases occur annually. You should keep your version up-to-date to provide the best Plaid Link experience in your application.
Update your project plugins
In your root-level (project-level) Gradle file (build.gradle
), add rules to include
the Android Gradle plugin. Check that you have Google's Maven repository as well.
1buildscript {2 repositories {3 // Check that you have the following line (if not, add it):4 google() // Google's Maven repository5 mavenCentral() // Include to import Plaid Link Android SDK6 }7 dependencies {8 // ...9 }10}
Add the PlaidLink SDK to your app
In your module (app-level) Gradle file (usually app/build.gradle
), add a line to the bottom of the file.
The latest version of the PlaidLink SDK is
and can be found on Maven Central.
1android {2 defaultConfig {3 minSdkVersion 21 // or greater4 }5}67dependencies {8 // ...9 implementation 'com.plaid.link:sdk-core:<insert latest version>'10}
Enable camera support (Identity Verification only)
If your app uses Identity Verification, a user may need to take a picture of identity documentation or a selfie during the Link flow. To support this workflow, the CAMERA
, WRITE_EXTERNAL_STORAGE
, RECORD_AUDIO
, and MODIFY_AUDIO_SETTINGS
permissions need to be added to your application's AndroidManifest.xml
. (While Plaid does not record any audio, some older Android devices require these last two permissions to use the camera.) The WRITE_EXTERNAL_STORAGE
permission should be limited to < Android 9 (i.e. maxSdk=28). If these permissions are not granted in an app that uses Identity Verification, the app may crash during Link.
Opening Link
Before you can open Link, you need to first create a link_token
by calling /link/token/create
from your backend.
This call should never happen directly from the mobile client, as it risks exposing your API secret.
The /link/token/create
call must include the android_package_name
parameter, which should match the applicationId
from your app-level
build.gradle
file. You can learn more about applicationId
in Google's Android
developer documentation.
1const request: LinkTokenCreateRequest = {2 user: {3 client_user_id: 'user-id',4 },5 client_name: 'Plaid Test App',6 products: ['auth', 'transactions'],7 country_codes: ['GB'],8 language: 'en',9 webhook: 'https://sample-web-hook.com',10 android_package_name: 'com.plaid.example'11 },12};13try {14 const response = await plaidClient.linkTokenCreate(request);15 const linkToken = response.data.link_token;16} catch (error) {17 // handle error18}
Create a LinkTokenConfiguration
Each time you open Link, you will need to get a new link_token
from your server and create a new
LinkTokenConfiguration
object with it.
1val linkTokenConfiguration = linkTokenConfiguration {2 token = "LINK_TOKEN_FROM_SERVER"3}
The Link SDK runs as a separate Activity
within your app. In order to return the result
to your app, it supports both the standard startActivityForResult
and onActivityResult
and the ActivityResultContract
result APIs.
Register a callback for an Activity Result
1private val linkAccountToPlaid =2registerForActivityResult(FastOpenPlaidLink()) {3 when (it) {4 is LinkSuccess -> /* handle LinkSuccess */5 is LinkExit -> /* handle LinkExit */6 }7}
Create a PlaidHandler
Create a PlaidHandler
- A PlaidHandler
is a one-time use object used to open a Link session.
It should be created as early as possible to warm up Link so that it opens quickly. We recommend
doing this as early as possible, since it must be completed before Link opens, and if you create
it just before opening Link, it can have a perceptible impact on Link startup time.
1val plaidHandler: PlaidHandler = 2 Plaid.create(application, linkTokenConfiguration)
Open Link
1linkAccountToPlaid.launch(plaidHandler)
At this point, Link will open, and will trigger the onSuccess
callback if the user successfully completes the Link flow.
onSuccess
The method is called when a user successfully links an Item. The onSuccess handler returns a LinkConnection
class that includes the public_token
, and additional Link metadata in the form of a LinkConnectionMetadata
class.
publicToken
metadata
accounts
accounts
will only include selected accounts.id
account_id
name
mask
subtype
type
verification_status
pending_automatic_verification
: The Item is pending automatic verificationpending_manual_verification
: The Item is pending manual micro-deposit verification. Items remain in this state until the user successfully verifies the deposit.automatically_verified
: The Item has successfully been automatically verifiedmanually_verified
: The Item has successfully been manually verifiedverification_expired
: Plaid was unable to automatically verify the deposit within 7 calendar days and will no longer attempt to validate the Item. Users may retry by submitting their information again through Link.verification_failed
: The Item failed manual micro-deposit verification because the user exhausted all 3 verification attempts. Users may retry by submitting their information again through Link.database_matched
: The Item has successfully been verified using Plaid's data sources.database_insights_pending
: The Database Insights result is pending and will be available upon Auth request.null
: Neither micro-deposit-based verification nor database verification are being used for the Item.institution
null
.name
'Wells Fargo'
institution_id
linkSessionId
metadataJson
1val success = result as LinkSuccess23// Send public_token to your server, exchange for access_token4val publicToken = success.publicToken5val metadata = success.metadata6metadata.accounts.forEach { account ->7 val accountId = account.id8 val accountName = account.name9 val accountMask = account.mask10 val accountSubType = account.subtype11}12val institutionId = metadata.institution?.id13val institutionName = metadata.institution?.name
onExit
The onExit
handler is called when a user exits Link without successfully linking an Item, or when an error occurs during Link initialization. The PlaidError
returned from the onExit
handler is meant to help you guide your users after they have exited Link. We recommend storing the error and metadata information server-side in a way that can be associated with the user. You’ll also need to include this and any other relevant information in Plaid Support requests for the user.
error
error
will be null
.displayMessage
null
if the error is not related to user action. This may change over time and is not safe for programmatic use.errorMessage
errorJson
LinkExitMetadata
linkSessionId
institution
null
.name
'Wells Fargo'
institution_id
status
requires_questions
requires_selections
requires_recaptcha
requires_code
choose_device
requires_credentials
requires_account _selection
institution_not_found
institution_not _supported
unknown
requestId
1val exit = result as LinkExit23val error = exit.error4error?.let { err ->5 val errorCode = err.errorCode6 val errorMessage = err.errorMessage7 val displayMessage = err.displayMessage8}9val metadata = exit.metadata10val institutionId = metadata.institution?.id11val institutionName = metadata.institution?.name12val linkSessionId = metadata.linkSessionId;13val requestId = metadata.requestId;
onEvent
The onEvent
callback is called at certain points in the Link flow. Unlike the handlers for onSuccess
and onExit
, the onEvent
handler is initialized as a global lambda passed to the Plaid
class. OPEN
, LAYER_READY
, and LAYER_NOT_AVAILABLE
events will be sent in real-time, and remaining events will be sent when the Link session is finished and onSuccess
or onExit
is called. Callback ordering is not guaranteed; onEvent
callbacks may fire before, after, or surrounding the onSuccess
or onExit
callback, and event callbacks are not guaranteed to fire in the order in which they occurred. If you need the exact time when an event happened, use the timestamp
property.
The following onEvent
callbacks are stable, which means that they are suitable for programmatic use in your application's logic: OPEN
, EXIT
, HANDOFF
, SELECT_INSTITUTION
, ERROR
, BANK_INCOME_INSIGHTS_COMPLETED
, IDENTITY_VERIFICATION_PASS_SESSION
, IDENTITY_VERIFICATION_FAIL_SESSION
, LAYER_READY
, LAYER_NOT_AVAILABLE
. The remaining callback events are informational and subject to change, and should be used for analytics and troubleshooting purposes only.
eventName
BANK_INCOME_INSIGHTS _COMPLETED
CLOSE_OAUTH
CONNECT_NEW _INSTITUTION
ERROR
error_code
metadata.FAIL_OAUTH
HANDOFF
IDENTITY_VERIFICATION _START_STEP
view_name
.IDENTITY_VERIFICATION _PASS_STEP
view_name
.IDENTITY_VERIFICATION _FAIL_STEP
view_name
.IDENTITY_VERIFICATION _PENDING_REVIEW_STEP
IDENTITY_VERIFICATION _CREATE_SESSION
IDENTITY_VERIFICATION _RESUME_SESSION
IDENTITY_VERIFICATION _PASS_SESSION
IDENTITY_VERIFICATION _FAIL_SESSION
IDENTITY_VERIFICATION _PENDING_REVIEW _SESSION
IDENTITY_VERIFICATION _OPEN_UI
IDENTITY_VERIFICATION _RESUME_UI
IDENTITY_VERIFICATION _CLOSE_UI
LAYER_NOT_AVAILABLE
LAYER_READY
open()
may now be called.MATCHED_SELECT _INSTITUTION
routing_number
was provided when calling /link/token/create
. To distinguish between the two scenarios, see LinkEventMetadata.match_reason
.MATCHED_SELECT_VERIFY _METHOD
OPEN
OPEN_MY_PLAID
OPEN_OAUTH
SEARCH_INSTITUTION
SKIP_SUBMIT_PHONE
SELECT_BRAND
SELECT_BRAND
event is only emitted for large financial institutions with multiple online banking portals.SELECT_DEGRADED _INSTITUTION
DEGRADED
health status and was shown a corresponding message.SELECT_DOWN _INSTITUTION
DOWN
health status and was shown a corresponding message.SELECT_FILTERED _INSTITUTION
SELECT_INSTITUTION
SUBMIT_ACCOUNT_NUMBER
account_number_mask
metadata to indicate the mask of the account number the user provided.SUBMIT_CREDENTIALS
SUBMIT_MFA
SUBMIT_PHONE
SUBMIT_ROUTING_NUMBER
routing_number
metadata to indicate user's routing number.TRANSITION_VIEW
TRANSITION_VIEW
event indicates that the user has moved from one view to the next.UPLOAD_DOCUMENTS
VERIFY_PHONE
VIEW_DATA_TYPES
UNKNOWN
UNKNOWN
event indicates that the event is not handled by the current version of the SDK.LinkEventMetadata
accountNumberMask
account_number_mask
is empty. Emitted by SUBMIT_ACCOUNT_NUMBER
.errorCode
ERROR
, EXIT
.errorMessage
ERROR
, EXIT
.errorType
ERROR
, EXIT
.exitStatus
EXIT
.institutionId
institutionName
institutionSearchQuery
SEARCH_INSTITUTION
.isUpdateMode
OPEN
.matchReason
returning_user
or routing_number
if emitted by: MATCHED_SELECT_INSTITUTION
.
Otherwise, this will be SAVED_INSTITUTION
or AUTO_SELECT_SAVED_INSTITUTION
if emitted by: SELECT_INSTITUTION
.routingNumber
SUBMIT_ROUTING_NUMBER
.linkSessionId
link_session_id
is a unique identifier for a single session of Link. It's always available and will stay constant throughout the flow. Emitted by: all events.mfaType
code
device
questions
selections
. Emitted by: SUBMIT_MFA
and TRANSITION_VIEW
when view_name
is MFA
.requestId
selection
selection
is used to describe selected verification method, then possible values are phoneotp
or password
; if selection
is used to describe the selected Auth Type Select flow, then possible values are flow_type_manual
or flow_type_instant
. Emitted by: MATCHED_SELECT_VERIFY_METHOD
and SELECT_AUTH_TYPE
.timestamp
2017-09-14T14:42:19.350Z
. Emitted by: all events.viewName
TRANSITION_VIEW
.ACCEPT_TOS
CONNECTED
CONSENT
CREDENTIAL
DATA_TRANSPARENCY
DATA_TRANSPARENCY _CONSENT
DOCUMENTARY _VERIFICATION
ERROR
EXIT
KYC_CHECK
LOADING
MATCHED_CONSENT
MATCHED_CREDENTIAL
MATCHED_MFA
MFA
NUMBERS
NUMBERS_SELECT _INSTITUTION
OAUTH
RECAPTCHA
RISK_CHECK
SCREENING
SELECT_ACCOUNT
SELECT_AUTH_TYPE
SELECT_BRAND
SELECT_INSTITUTION
SELECT_SAVED_ACCOUNT
SELECT_SAVED _INSTITUTION
SELFIE_CHECK
SUBMIT_PHONE
UPLOAD_DOCUMENTS
VERIFY_PHONE
VERIFY_SMS
metadataJson
1Plaid.setLinkEventListener { event -> Log.i("Event", event.toString()) }
Submit
The submit
function is currently only used in the Layer product. It allows the client application to submit additional user-collected data to the Link flow (e.g. a user phone number).
submissionData
phoneNumber
1val submissionData = SubmissionData(phoneNumber)2plaidHandler.submit(submissionData)
Upgrading
The latest version of the SDK is available from GitHub. New versions of the SDK are released frequently. Major releases occur annually. The Link SDK uses Semantic Versioning, ensuring that all non-major releases are non-breaking, backwards-compatible updates. We recommend you update regularly (at least once a quarter, and ideally once a month) to ensure the best Plaid Link experience in your application.
SDK versions are supported for two years; with each major SDK release, Plaid will stop officially supporting any previous SDK versions that are more than two years old. While these older versions are expected to continue to work without disruption, Plaid will not provide assistance with unsupported SDK versions.
Next steps
If you run into problems integrating with Plaid Link on Android, see Troubleshooting the Plaid Link Android SDK.
Once you've gotten Link working, see Link best practices for recommendations on further improving the Link flow.