Multi-Item Link
Allow end users to connect accounts from multiple institutions in a single Link session
Overview
Multi-Item Link allows end users to add multiple Items in the same Link session. This flow is designed for contexts where you expect the user to link multiple accounts; for example, to provide a complete financial picture for a lending or personal finance use case.
Multi-Item Link can result in more Items being connected per user, due to reduced friction, and can simplify your app's logic and user experience.
Multi-Item Link is compatible with the following Plaid products: Auth, Transactions, Liabilities, Investments, Income, Assets, and Plaid Check Consumer Reports (including Income and Partner Insights). It does not require special enablement and is automatically available to all customers.
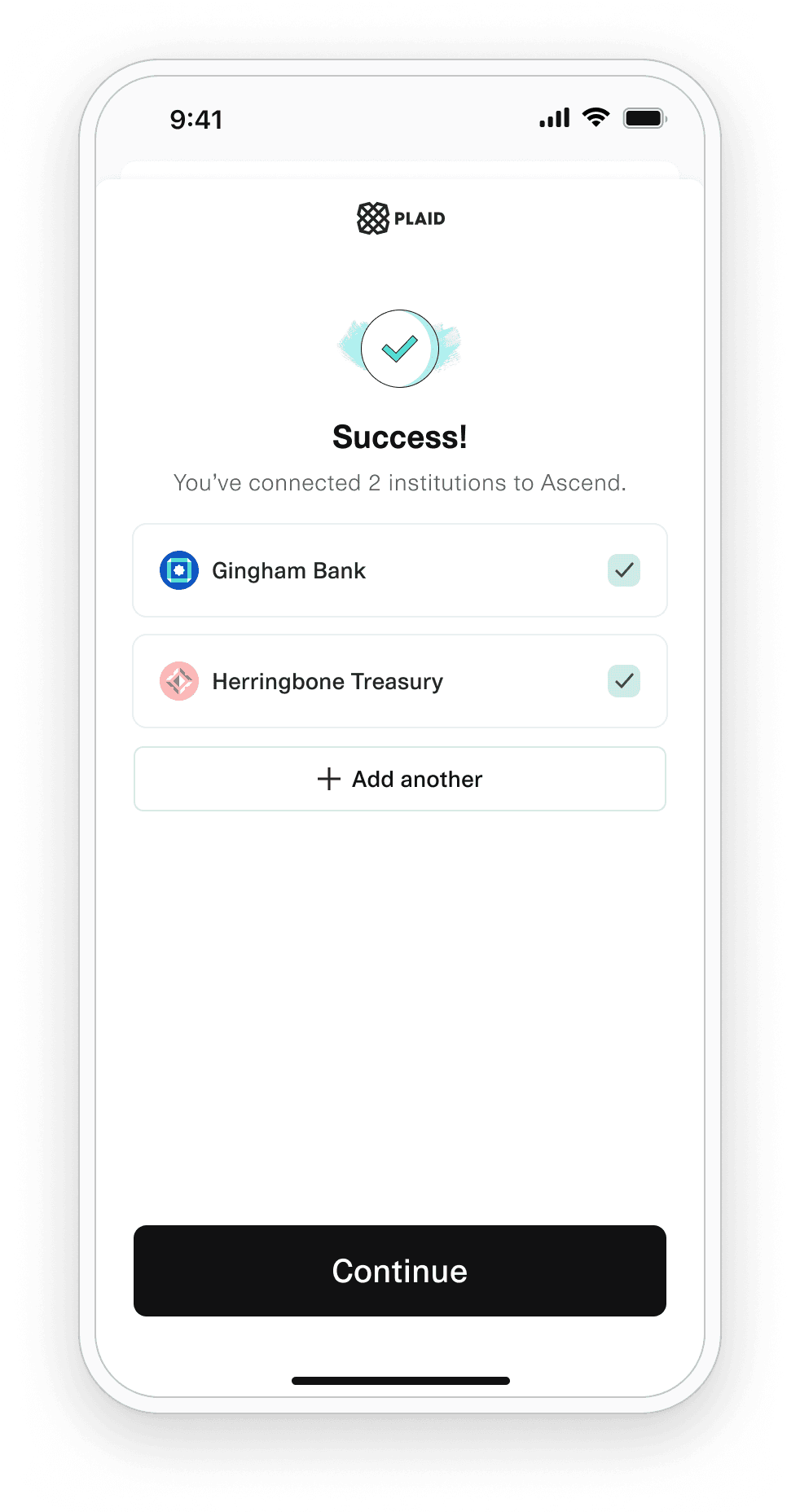
Integration process
Creating a Link token
First, create a user token using the /user/create
endpoint.
1// client_user_id is your own internal identifier for the end user2{3 "client_id": "${PLAID_CLIENT_ID}",4 "secret": "${PLAID_SECRET}",5 "client_user_id" : "c0e2c4ee-b763-4af5-cfe9-46a46bce883d" 6}
Next, call /link/token/create
with the user_token
you just created, and the enable_multi_item_link
field set to true
.
1// Sample link/token/create request2{3 "client_id": "${PLAID_CLIENT_ID}",4 "secret": "${PLAID_SECRET}",5 "client_name" : "Plaid Test App",6 "user_token": "user-sandbox-b0e2c4ee-a763-4df5-bfe9-46a46bce9",7 "enable_multi_item_link": true,8 "user": {9 "client_user_id": "c0e2c4ee-b763-4af5-cfe9-46a46bce883d",10 },11 "products": ["transactions"],12 "country_codes": ["US"],13 "language": "en",14}
Once you have created a Link token, you will launch Link as normal, according to the standard instructions for your platform.
Obtaining a public token
In most other Plaid integration methods, upon completion of the Link flow, you will receive a public token via the onSuccess
callback. In a Multi-Item Link session, the onSuccess
callback will be empty, and you will instead receive information about the Link flow (including the public_token
array) via the SESSION_FINISHED
webhook.
When the entire Multi-Item Link flow is complete and the user has exited Link, Plaid will fire a SESSION_FINISHED
webhook that contains information about what caused the session to end, as well as the public tokens if the session completed successfully.
1{2 "webhook_type": "LINK",3 "webhook_code": "SESSION_FINISHED",4 "status": "SUCCESS",5 "link_session_id": "356dbb28-7f98-44d1-8e6d-0cec580f3171",6 "link_token": "link-sandbox-af1a0311-da53-4636-b754-dd15cc058176",7 "public_tokens": [8 "public-sandbox-b0e2c4ee-a763-4df5-bfe9-46a46bce993d"9 ],10 "environment": "sandbox"11}
If you want to start getting results as soon as each Item is added, you can listen for the ITEM_ADD_RESULT
webhook, which will fire after each completed Item add within the Link session.
1{2 "webhook_type": "LINK",3 "webhook_code": "ITEM_ADD_RESULT",4 "link_session_id": "356dbb28-7f98-44d1-8e6d-0cec580f3171",5 "link_token": "link-sandbox-af1a0311-da53-4636-b754-dd15cc058176",6 "public_token": "public-sandbox-b0e2c4ee-a763-4df5-bfe9-46a46bce993d",7 "environment": "sandbox"8}
Detailed session data, including the public tokens, will also be available from /link/token/get
for six hours after the session has completed. In general, it is recommended to use the webhook to obtain the public token, since it will allow you to get the public token more promptly, but you may want to use /link/token/get
if your integration does not use webhooks, or as a backup mechanism if your system missed webhooks due to an outage.
When using Multi-Item Link, make sure to obtain the public_token
from either the SESSION_FINISHED
webhook, the ITEM_ADD_RESULT
webhook, or the results.item_add_results
object returned by /link/token/get
. The on_success
object returned by /link/token/get
is deprecated and should not be used to obtain the public token in Multi-Item Link flows, as it will contain only one public token, rather than all of the public tokens from the session.
Frontend changes
When using Multi-Item Link, the frontend onSuccess
callback will be empty, but other callbacks, such as onExit
and onEvent
, will remain populated as usual. Frontend callbacks can still be used to signal the end of the Link session.
Multi-Item Link with multiple products
If you are using Multi-Item Link, the same Link token settings will be used for every Item in the Link flow. This means, for example, that Auth with Same Day Micro-deposits cannot be used in the same Multi-Item Link flow as Income, because Auth must be initialized by itself to be used with Same Day Micro-deposits.
Using Auth or Transfer with Multi-Item Link
A common use case for including Auth or Transfer as part of a Multi-Item link process is when you want one of the linked accounts to be used for sending or receiving payments. For example, as a lender, you may want to disburse the user's loan to a linked account, or as a financial management app, you may want to use one of the linked accounts to collect a monthly subscription fee.
In this situation, your app will want to connect to multiple institutions, but only need one account for payment purposes. For this use case, the recommended flow is:
Put
auth
ortransfer
in theoptional_products
array when calling/link/token/create
; this will request Auth or Transfer permissions when possible, but will not block linking accounts that don't support Auth and won't cause you to be billed for Auth unless you use an Auth endpoint on the Item.Locate eligible accounts by looking at the
/link/token/get
response object and traversinglink_sessions.results.item_add_results[].account[].subtype
to check each account on each Item, to see if it is of subtypechecking
orsavings
.For each Item on an account where the subtype is
checking
orsavings
, call/item/get
and check theitem.products[]
array forauth
.If the account subtype is
checking
orsavings
, and theitem.products[]
value contains"auth"
as a value, then the account is eligible for use with Plaid Auth or Transfer. Present a UI in which the user can select an eligible account to use for sending or receiving funds. To avoid confusion, it is recommended that you present this UI even if there is only one eligible account.(Optional) You can add a button to this UI to link a different account to use for funds transfer, and have that button launch Link with just
auth
ortransfer
, which could allow the end user to link a payment account via a non-credential-based flow, such as Same Day Micro-Deposits or Database Insights.Once the user has selected an account to use for payments, call the appropriate endpoint, such as
/auth/get
,/processor/token/create
, or/transfer/authorization/create
, on the corresponding Item.