Link React Native SDK
Reference for integrating with the Link React Native SDK
This guide covers the latest major version of the Link React Native SDK, which is version 12.x.x. For information on migrating from older versions, see Migration guides.
Overview
Prefer to learn with code examples? A GitHub repo showing a working example Link implementation is available for this topic.
To get started with Plaid Link for React Native youʼll want to sign up for free API keys through the Plaid Dashboard.
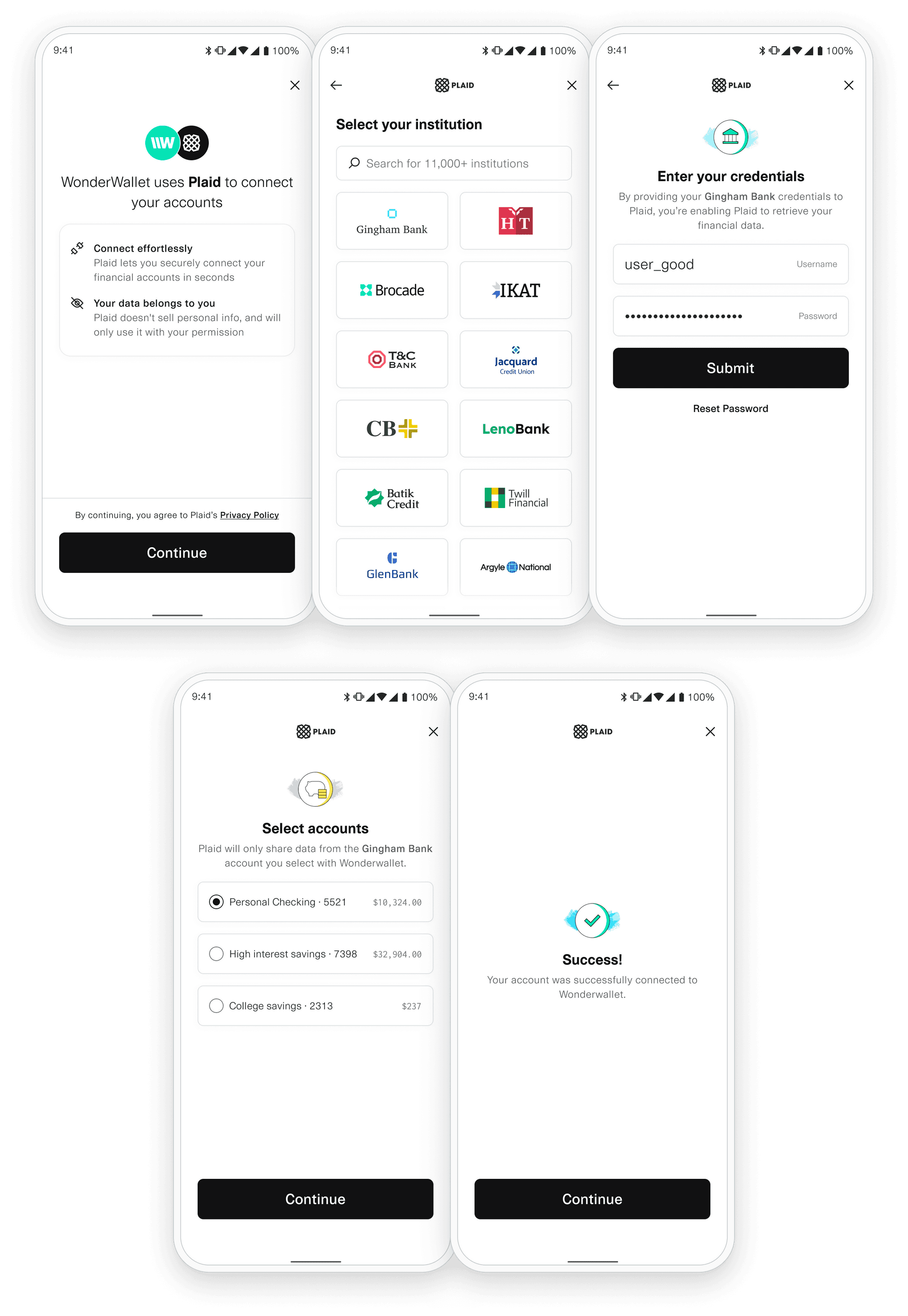
Requirements
- React Native Version
0.66.0
or higher
New versions of the React Native SDK are released frequently. Major releases occur annually. The Link SDK uses Semantic Versioning, ensuring that all non-major releases are non-breaking, backwards-compatible updates. We recommend you update regularly (at least once a quarter, and ideally once a month) to ensure the best Plaid Link experience in your application.
SDK versions are supported for two years; with each major SDK release, Plaid will stop officially supporting any previous SDK versions that are more than two years old. While these older versions are expected to continue to work without disruption, Plaid will not provide assistance with unsupported SDK versions.
Version Compatibility
React Native SDK | Android SDK | iOS SDK | Status |
---|---|---|---|
12.x.x | 5.0+ | >=6.0.0 | Active, supports Xcode 16 |
11.x.x | 4.1.1+ | >=5.1.0 | Active, supports Xcode 15 |
10.x.x | 3.10.1+ | >=4.1.0 | Active, supports Xcode 14 |
9.x.x | 3.10.1+ | >=4.1.0 | Deprecated, supports Xcode 14 |
Getting Started
Installing the SDK
In your react-native project directory, run:
1npm install --save react-native-plaid-link-sdk
iOS Setup
Add Plaid to your project's Podfile as follows (likely located at ios/Podfile).
1pod 'Plaid', '~> <insert latest version>'
Autolinking should install the CocoaPods dependencies for iOS project. If it fails you can run
1cd ios && bundle install && bundle exec pod install
Android Setup
Requirements:
- Android 5.0 (API level 21) and above.
- Your compileSdkVersion must be 35.
- Android gradle plugin 4.x and above.
Autolinking should handle all of the Android setup.
- Register your Android package name in the Dashboard. This is required in order to connect to OAuth institutions (which includes most major banks).
Sample app
For a sample app that demonstrates a minimal integration with the React Native Plaid Link SDK, see the Tiny Quickstart (React Native).
Opening Link
Before you can open Link, you need to first create a link_token
. A link_token
can be configured for
different Link flows and is used to control much of Link's behavior. To see how to create a new
link_token
, see the API Reference entry for /link/token/create
.
If your React Native application will be used on Android, the link/token/create
call should include the android_package_name
parameter.
Each time you open Link, you will need to get a new link_token
from your server.
Next, open Link via the create
and open
methods. These functions require version 11.6 or later of the Plaid React Native SDK.
If using a version earlier than 11.6, you must open Link with the legacy PlaidLink
component, which configures Link and registers a callback in a single component. This approach has higher user-facing latency than using the create
and open
methods.
create
You can initiate the Link preloading process by invoking the create
function. After calling create
, call open
to open Link. This function requires SDK version 11.6 or later.
linkTokenConfiguration
token
link_token
to be used to authenticate your app with Link. The link_token
is created by calling /link/token/create
and is a short lived, one-time use token that should be unique for each Link session. In addition to the primary flow, a link_token
can be configured to launch Link in update mode. See the /link/token/create
endpoint for a full list of configurations.noLoadingState
logLevel
DEBUG
, INFO
, WARN
, ERROR
1<TouchableOpacity2 style={styles.button}3 onPress={() => {4 create({token: linkToken});5 setDisabled(false);6 }7 }>8 <Text style={styles.button}>Create Link</Text>9</TouchableOpacity>
open
After calling create
, you can subsequently invoke the open
function. Note that maximizing the delay between these two calls will reduce latency for your users by allowing Link more time to load. This function requires SDK version 11.6 or later.
iosPresentationStyle
MODAL
.MODAL
, FULL_SCREEN
logLevel
DEBUG
, INFO
, WARN
, ERROR
1<TouchableOpacity2 disabled={disabled}3 style={disabled ? styles.disabledButton : styles.button}4 onPress={() => {5 const openProps = {6 onSuccess: (success: LinkSuccess) => {7 console.log(success);8 },9 onExit: (linkExit: LinkExit) => {10 console.log(exit);11 };12 open(openProps);13 setDisabled(true);14 }}>15 <Text style={styles.button}>Open Link</Text>16</TouchableOpacity>
PlaidLink
PlaidLink is a React component used to open Link from a React Native application. PlaidLink renders a Pressable component, which wraps the component you provide and intercepts onPress events to open Link. PlaidLink is an older alternative to the create
and open
methods, which offer reduced Link latency and improved performance.
tokenConfig
token
link_token
to be used to authenticate your app with Link. The link_token
is created by calling /link/token/create
and is a short lived, one-time use token that should be unique for each Link session. In addition to the primary flow, a link_token
can be configured to launch Link in update mode. See the /link/token/create
endpoint for a full list of configurations.logLevel
DEBUG
, INFO
, WARN
, ERROR
extraParams
children
1<PlaidLink2 tokenConfig={{3 token: '#GENERATED_LINK_TOKEN#',4 }}5 onSuccess={(success: LinkSuccess) => {6 console.log(success);7 }}8 onExit={(exit: LinkExit) => {9 console.log(exit);10 }}11>12 <Text>Add Account</Text>13</PlaidLink>
onSuccess
The method is called when a user successfully links an Item. The onSuccess handler returns a LinkConnection
class that includes the public_token
, and additional Link metadata in the form of a LinkConnectionMetadata
class.
publicToken
null
. If using Document Income or Payroll Income, the public_token
will be returned, but is not used.metadata
accounts
accounts
will only include selected accounts.id
account_id
name
mask
type
subtype
verification_status
pending_automatic_verification
pending_manual_verification
automatically_verified
manually_verified
verification_expired
verification_failed
database_matched
database_insights_pending
null
institution
null
.linkSessionId
metadataJson
1const onSuccess = (success: LinkSuccess) => {2 // If using Item-based products, exchange public_token3 // for access_token4 fetch('https://yourserver.com/exchange_public_token', {5 method: 'POST',6 headers: {7 "Content-Type": "application/json",8 },9 body: JSON.Stringify({10 publicToken: linkSuccess.publicToken,11 accounts: linkSuccess.metadata.accounts,12 institution: linkSuccess.metadata.institution,13 linkSessionId: linkSuccess.metadata.linkSessionId,14 }),15 });16};
onExit
The onExit
handler is called when a user exits Link without successfully linking an Item, or when an error occurs during Link initialization. The PlaidError
returned from the onExit
handler is meant to help you guide your users after they have exited Link. We recommend storing the error and metadata information server-side in a way that can be associated with the user. You’ll also need to include this and any other relevant info in Plaid Support requests for the user.
error
error
will be null
.displayMessage
null
if the error is not related to user action. This may change over time and is not safe for programmatic use.errorCode
errorType
has a specific set of errorCodes
. A code of 499 indicates a client-side exception.errorType
errorMessage
errorJson
metadata
linkSessionId
institution
null
.status
requires_questions
requires_selections
requires_recaptcha
requires_code
choose_device
requires_credentials
requires_account_selection
requires_oauth
institution_not_found
institution_not_supported
unknown
requestId
metadataJson
1const onExit = (linkExit: LinkExit) => {2 supportHandler.report({3 error: linkExit.error,4 institution: linkExit.metadata.institution,5 linkSessionId: linkExit.metadata.linkSessionId,6 requestId: linkExitlinkExit.metadata.requestId,7 status: linkExit.metadata.status,8 });9};
onEvent
The React Native Plaid module emits onEvent
events throughout the account linking process.
To receive these events use the usePlaidEmitter
hook.
The onEvent
callback is called at certain points in the Link flow. Unlike the handlers for onSuccess
and onExit
, the onEvent
handler is initialized as a global lambda passed to the Plaid
class. OPEN
, LAYER_READY
, and LAYER_NOT_AVAILABLE
events will be sent immediately in real-time, and remaining events will be sent when the Link session is finished and onSuccess
or onExit
is called. Callback ordering is not guaranteed; onEvent
callbacks may fire before, after, or surrounding the onSuccess
or onExit
callback, and event callbacks are not guaranteed to fire in the order in which they occurred. If you need the exact time when an event happened, use the timestamp
property.
The following onEvent
callbacks are stable, which means that they are suitable for programmatic use in your application's logic: OPEN
, EXIT
, HANDOFF
, SELECT_INSTITUTION
, ERROR
, BANK_INCOME_INSIGHTS_COMPLETED
, IDENTITY_VERIFICATION_PASS_SESSION
, IDENTITY_VERIFICATION_FAIL_SESSION
, LAYER_READY
, LAYER_NOT_AVAILABLE
. The remaining callback events are informational and subject to change, and should be used for analytics and troubleshooting purposes only.
eventName
AUTO_SUBMIT_PHONE
/link/token/create
call and the user has previously consented to receive OTP codes from Plaid.BANK_INCOME_INSIGHTS_COMPLETED
CLOSE_OAUTH
CONNECT_NEW_INSTITUTION
ERROR
error_code
metadata.FAIL_OAUTH
HANDOFF
IDENTITY_VERIFICATION_START_STEP
view_name
.IDENTITY_VERIFICATION_PASS_STEP
view_name
.IDENTITY_VERIFICATION_FAIL_STEP
view_name
.IDENTITY_VERIFICATION_PENDING_REVIEW_STEP
IDENTITY_VERIFICATION_CREATE_SESSION
IDENTITY_VERIFICATION_RESUME_SESSION
IDENTITY_VERIFICATION_PASS_SESSION
IDENTITY_VERIFICATION_PENDING_REVIEW_SESSION
IDENTITY_VERIFICATION_FAIL_SESSION
IDENTITY_VERIFICATION_OPEN_UI
IDENTITY_VERIFICATION_RESUME_UI
IDENTITY_VERIFICATION_CLOSE_UI
LAYER_NOT_AVAILABLE
LAYER_READY
open()
may now be called.MATCHED_SELECT_INSTITUTION
routing_number
was provided when calling /link/token/create
. For details on which scenario is triggering the event, see metadata.matchReason
.MATCHED_SELECT_VERIFY_METHOD
OPEN
OPEN_MY_PLAID
OPEN_OAUTH
SEARCH_INSTITUTION
SELECT_BRAND
SELECT_BRAND
event is only emitted for large financial institutions with multiple online banking portals.SELECT_DEGRADED_INSTITUTION
DEGRADED
health status and was shown a corresponding message.SELECT_DOWN_INSTITUTION
DOWN
health status and was shown a corresponding message.SELECT_FILTERED_INSTITUTION
SELECT_INSTITUTION
SKIP_SUBMIT_PHONE
SUBMIT_ACCOUNT_NUMBER
account_number_mask
metadata to indicate the mask of the account number the user provided.SUBMIT_CREDENTIALS
SUBMIT_MFA
SUBMIT_OTP
SUBMIT_PHONE
SUBMIT_ROUTING_NUMBER
routing_number
metadata to indicate user's routing number.TRANSITION_VIEW
TRANSITION_VIEW
event indicates that the user has moved from one view to the next.VERIFY_PHONE
VIEW_DATA_TYPES
UNKNOWN
UNKNOWN
event indicates that the event is not handled by the current version of the SDK.metadata
submitAccountNumber
account_number_mask
is empty. Emitted by SUBMIT_ACCOUNT_NUMBER
.errorCode
ERROR
, EXIT
.errorMessage
ERROR
, EXIT
.errorType
ERROR
, EXIT
.exitStatus
EXIT
.institutionId
institutionName
institutionSearchQuery
SEARCH_INSTITUTION
.isUpdateMode
OPEN
.matchReason
returning_user
or routing_number
if emitted by: MATCHED_SELECT_INSTITUTION
.
Otherwise, this will be SAVED_INSTITUTION
or AUTO_SELECT_SAVED_INSTITUTION
if emitted by: SELECT_INSTITUTION
.routingNumber
SUBMIT_ROUTING_NUMBER
.linkSessionId
linkSessionId
is a unique identifier for a single session of Link. It's always available and will stay constant throughout the flow. Emitted by: all events.mfaType
code
device
questions
selections
. Emitted by: SUBMIT_MFA
and TRANSITION_VIEW
when view_name
is MFA
.requestId
selection
selection
is used to describe selected verification method, then possible values are phoneotp
or password
; if selection
is used to describe the selected Auth Type Select flow, then possible values are flow_type_manual
or flow_type_instant
. Emitted by: MATCHED_SELECT_VERIFY_METHOD
and SELECT_AUTH_TYPE
.timestamp
2017-09-14T14:42:19.350Z
. Emitted by: all events.viewName
TRANSITION_VIEW
.ACCEPT_TOS
CONNECTED
CONSENT
CREDENTIAL
DATA_TRANSPARENCY
DATA_TRANSPARENCY_CONSENT
DOCUMENTARY_VERIFICATION
ERROR
EXIT
INSTANT_MICRODEPOSIT_AUTHORIZED
KYC_CHECK
LOADING
MATCHED_CONSENT
MATCHED_CREDENTIAL
MATCHED_MFA
MFA
NUMBERS
NUMBERS_SELECT_INSTITUTION
OAUTH
RECAPTCHA
RISK_CHECK
SAME_DAY_MICRODEPOSIT_AUTHORIZED
SCREENING
SELECT_ACCOUNT
SELECT_AUTH_TYPE
SELECT_BRAND
SELECT_INSTITUTION
SELECT_SAVED_ACCOUNT
SELECT_SAVED_INSTITUTION
SELFIE_CHECK
SUBMIT_PHONE
UPLOAD_DOCUMENTS
VERIFY_PHONE
VERIFY_SMS
1usePlaidEmitter((event) => {2 console.log(event);3});
submit
The submit
function is currently only used in the Layer product. It allows the client application to submit additional user-collected data to the Link flow (e.g. a user phone number).
submissionData
phoneNumber
1submit({2 "phone_number": "+14155550123"3})
destroy
The destroy
function clears state and resources from a previously opened session. The destroy
function is only available on Android, as this state clearing behavior occurs automatically on iOS. destroy
is intended for use with the Layer product and should be used if you are making multiple calls to create
before calling submit
. By calling destroy
between the create
calls, you can avoid unexpected behavior on the submit
call.
1create(tokenConfiguration1)2async () => {3 try {4 await destroy(); // Clear previous session state5 create(tokenConfiguration2);6 submit(phoneNumber);7 } catch (e) {8 console.error('Error during flow:', e);9 }10}
OAuth
Using Plaid Link with an OAuth flow requires some additional setup instructions. For details, see the OAuth guide.
Upgrading
The latest version of the SDK is available from GitHub. New versions of the SDK are released frequently. Major releases occur annually. The Link SDK uses Semantic Versioning, ensuring that all non-major releases are non-breaking, backwards-compatible updates. We recommend you update regularly (at least once a quarter, and ideally once a month) to ensure the best Plaid Link experience in your application.
SDK versions are supported for two years; with each major SDK release, Plaid will stop officially supporting any previous SDK versions that are more than two years old. While these older versions are expected to continue to work without disruption, Plaid will not provide assistance with unsupported SDK versions.
Migration guides
- Version 12.x removes the
PlaidLink
component andopenLink
function, which were deprecated in version 11.6.0. If you are using this method of opening Link, replace it with the new process that usescreate
and thenopen
. For details, see Opening Link the README. Version 12.x also removes thePROFILE_ELIGIBILITY_CHECK_ERROR
. - Version 12.x updates the Android target SDK version and compile version from 33 to 35 and requires use of an Xcode 16 toolchain.
- Version 11.x contains several breaking changes from previous major versions. For details, see the migration guide on GitHub.
- When upgrading from 9.x to 10.x or later, you should remove any invocation of
useDeepLinkRedirector
on iOS, as it has been removed from the SDK, since it is no longer required for handling Universal Links. You must make sure you are using a compatible version of React Native (0.66.0 or higher) and the Plaid iOS SDK (see the version compatibility chart on GitHub). - No code changes are required to upgrade from 8.x to 9.x, although you must make sure you are using a compatible version of the Plaid iOS SDK (4.1.0 or higher).
- The only difference between version 7.x and 8.x is that 8.x adds support for Xcode 14. No code changes are required to upgrade from 7.x to 8.x, although you must convert to an Xcode 14 toolchain.