Add Stripe to your app
Use Stripe with Plaid Auth to send and receive payments

Plaid and Stripe have partnered to offer frictionless money transfers without the need to ever handle an account or routing number. Use Plaid Link to instantly authenticate your customer's account and generate a Stripe bank account token so that you can accept ACH payments via Stripe.
Plaid can be used with Stripe's ACH Charges and Sources API as well as with the newer Stripe Payment Intents API. Note that Stripe has marked the Charges and Sources API as deprecated. However, the Charges and Sources API and Plaid integration are still both fully supported by both Plaid and Stripe. To use Plaid with the Payment Intents API, you will need to contact Stripe to request a manual exception.
This guide is designed for those who already have developer accounts with both Stripe and Plaid. If that's not you, Sign up for a Plaid Dashboard account, then head over to the Stripe docs to get a Stripe account.
The sample Plaid integration code used in the Stripe-hosted Charges and Sources ACH documentation demonstrates the use of a legacy version of the Plaid client libraries. For new customers, we recommend using the sample code within this guide for building your integration.
Getting Started
You'll first want to familiarize yourself with Plaid Link, a drop-in client-side integration for the Plaid API that handles input validation, error handling, and multi-factor authentication.
Your customers will use Link to authenticate with their financial institution and select the depository account they wish to use for ACH transactions. From there, you'll receive a Plaid access_token
, allowing you to leverage real-time balance checks and transaction data, and a Stripe bank_account_token
, which allows you to move money via Stripe's ACH API without ever handling an account or routing number.
Instructions
Set up your Plaid and Stripe accounts
You'll need accounts at both Plaid and Stripe in order to use the Plaid Link + Stripe integration. You'll also need to connect your Plaid and Stripe accounts so that Plaid can facilitate the creation of bank account tokens on your behalf.
First, sign up for a Stripe account if you do not already have one and then verify that it is enabled for ACH access. To verify that your Stripe account is ACH enabled, head to the Charges and Sources ACH Guide when you are logged in to your Stripe account. If you see:
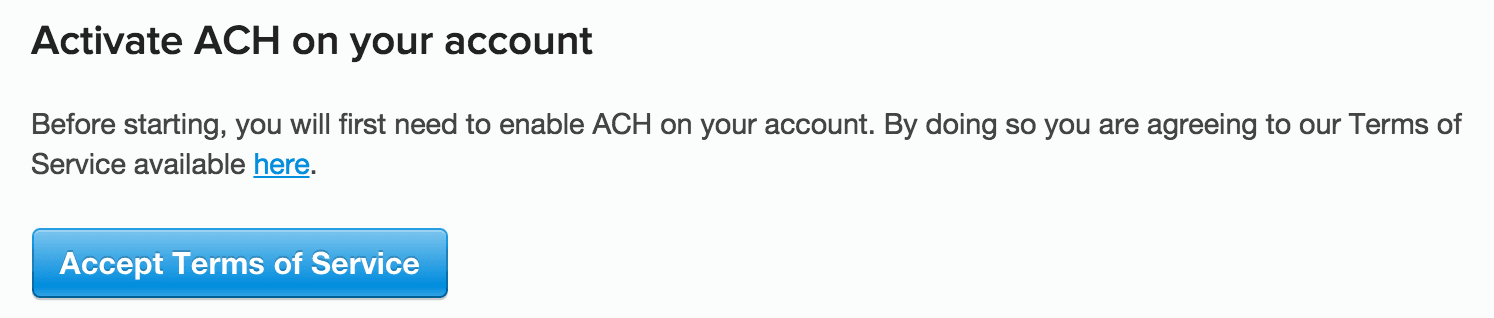
your account is not enabled. Click 'Accept Terms of Service' to enable your Stripe account for ACH. If you do not see the 'Accept Terms of Service' button, your Stripe account is already enabled for ACH access and you do not need to take any action.
Next, verify that your Plaid account is enabled for the integration. If you do not have a Plaid account, create one. Your account will be automatically enabled for integration access.
To verify that your Plaid account is enabled for the integration, go to the Integrations section of the account dashboard. If you see:
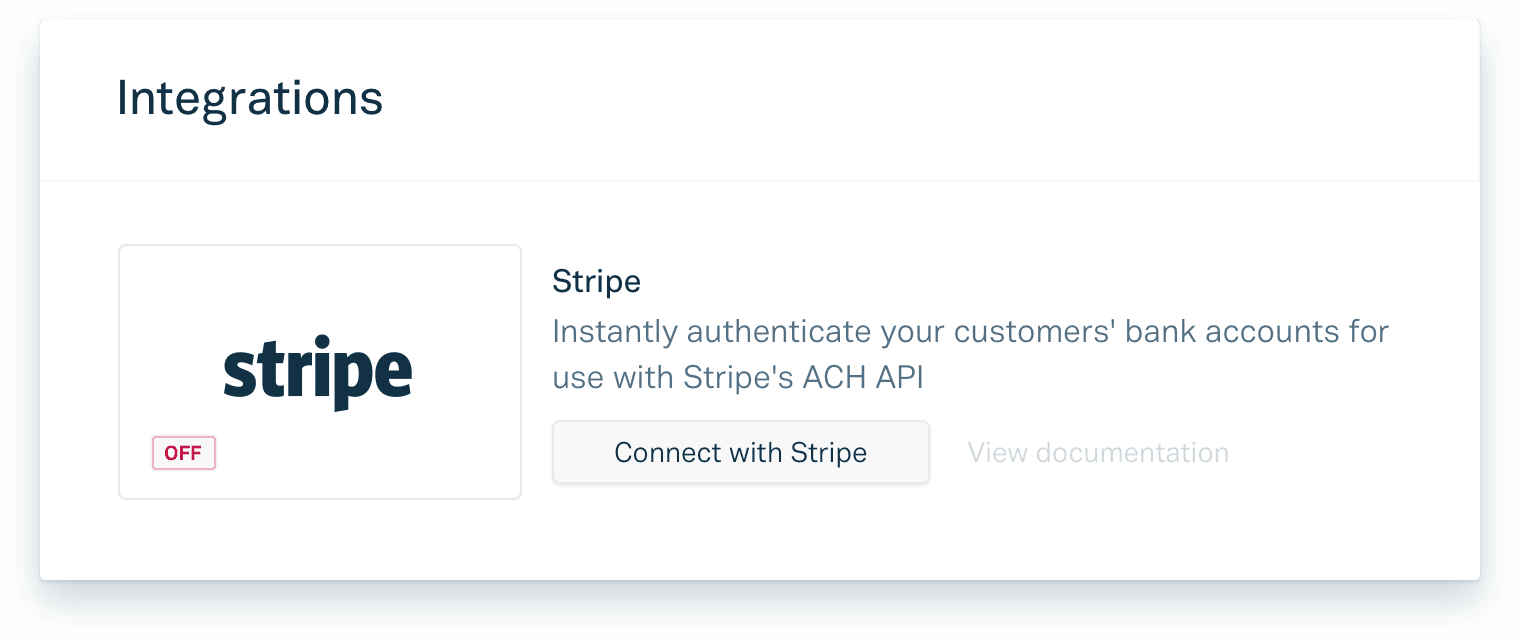
your Plaid account is enabled for the integration but you have not connected your Stripe account.
Click the 'Connect With Stripe' button to connect your Plaid and Stripe accounts. This step is required so that Plaid can facilitate the creation of Stripe bank account tokens on your behalf.
Once your Stripe account is connected, you'll see:
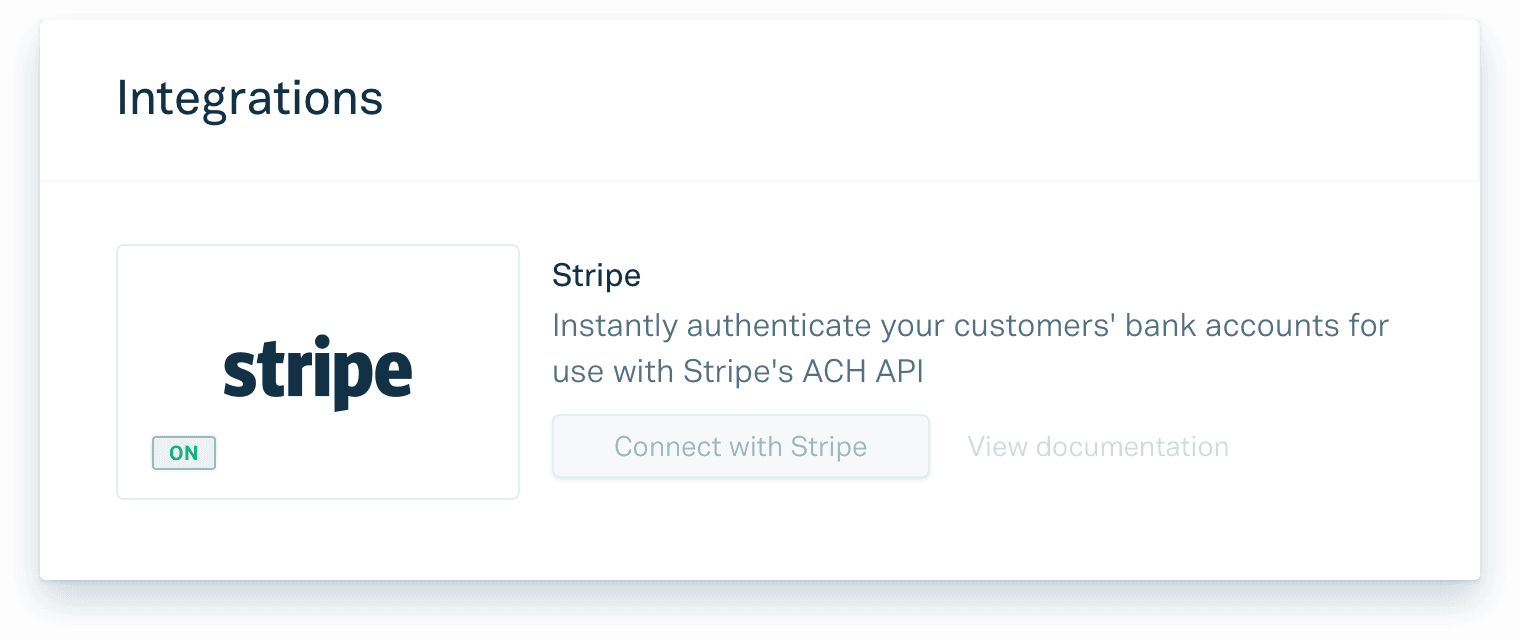
Your Plaid account is now set up for the integration!
Complete your Plaid application profile and company profile
After connecting your Stripe and Plaid accounts, you'll need to complete your Plaid application profile and company profile in the Dashboard, which involves filling out basic information about your app, such as your company name and website. This step helps your end-users learn more how your product uses their bank information and is also required for connecting to some banks.
Create a link_token
In order to integrate with Plaid Link, you will first need to create a link_token
. A link_token
is a short-lived, one-time use token that is used to authenticate your app with Link. To create one,
make a /link/token/create
request with your client_id
, secret
, and a few other
required parameters from your app server. For a full list of parameters, see the /link/token/create
reference.
To see your client_id
and secret
, visit the Plaid Dashboard.
1app.post('/api/create_link_token', async function (request, response) {2 // Get the client_user_id by searching for the current user3 const user = await User.find(...);4 const clientUserId = user.id;5 const request = {6 user: {7 // This should correspond to a unique id for the current user.8 client_user_id: clientUserId,9 },10 client_name: 'Plaid Test App',11 products: ['auth'],12 language: 'en',13 webhook: 'https://webhook.example.com',14 redirect_uri: 'https://domainname.com/oauth-page.html',15 country_codes: ['US'],16 };17 try {18 const createTokenResponse = await client.linkTokenCreate(request);19 response.json(createTokenResponse.data);20 } catch (error) {21 // handle error22 }23});
Integrate with Plaid Link
Once you have a link_token
, all it takes is a few lines of client-side JavaScript to launch Link.
Then, in the onSuccess
callback, you can call a simple server-side handler to exchange the Link public_token
for a Plaid access_token
and a Stripe bank account token.
1<button id="linkButton">Open Link - Institution Select</button>2<script src="https://cdn.plaid.com/link/v2/stable/link-initialize.js"></script>3<script>4 (async function(){5 var linkHandler = Plaid.create({6 // Make a request to your server to fetch a new link_token.7 token: (await $.post('/create_link_token')).link_token,8 onLoad: function() {9 // The Link module finished loading.10 },11 onSuccess: function(public_token, metadata) {12 // The onSuccess function is called when the user has13 // successfully authenticated and selected an account to14 // use.15 //16 // When called, you will send the public_token17 // and the data about which account to use18 // to your backend app server. In this example,19 // we assume "Account Select" is set to20 // "enabled for one account".21 //22 // sendDataToBackendServer({23 // public_token: public_token,24 // account_id: metadata.accounts[0].id25 // });26 },27 onExit: function(err, metadata) {28 // The user exited the Link flow.29 if (err != null) {30 // The user encountered a Plaid API error31 // prior to exiting.32 }33 // metadata contains information about the institution34 // that the user selected and the most recent35 // API request IDs.36 // Storing this information can be helpful for support.37 },38 });39 })();40
41 // Trigger the authentication view42 document.getElementById('linkButton').onclick = function() {43 linkHandler.open();44 };45</script>
See the Link parameter reference for complete documentation on possible configurations.
Plaid.create
accepts one argument, a configuration Object
, and returns an Object
with three functions, open
, exit
, and destroy
. Calling open
will display the "Institution Select" view, calling exit
will close Link, and calling destroy
will clean up the iframe.
Write server-side handler
The Link module handles the entire onboarding flow securely and quickly, but does not actually retrieve account data for a user. Instead, the Link module returns a public_token
and an accounts
array, which is a property on the metadata
object, via the onSuccess
callback. Exchange this public_token
for a Plaid access_token
using the /item/public_token/exchange
API endpoint.
The accounts
array will contain information about bank accounts associated with the credentials entered by the user, and may contain multiple accounts if the user has more than one bank account at the institution. In order to avoid any confusion about which account your user wishes to use with Stripe, it is recommended to set Account Select to "enabled for one account" in the Plaid Dashboard. When this setting is selected, the accounts
array will always contain exactly one element.
Once you have identified the account you will use, you will call /processor/stripe/bank_account_token/create
, sending Plaid the account_id
property of the account along with the access_token
you obtained from /item/public_token/exchange
. Plaid will create and return a Stripe bank account token for this account, which can then be used to move money via Stripe's ACH API. The bank account token will be linked to the Stripe account you linked in your Plaid Dashboard.
Note that the Stripe bank account token is a one-time use token. To store bank account information for later use, you can use a Stripe customer object and create an associated bank account from the token, or you can use a Stripe Custom account and create an associated external bank account from the token. This bank account information should work indefinitely, unless the user's bank account information changes or they revoke Plaid's permissions to access their account (when using certain financial institutions). The Plaid access_token
, on the other hand, does not expire and should be persisted securely.
Stripe bank account information cannot be modified once the bank account token has been created. If you ever need to change the bank account details used by Stripe for a specific customer, you should repeat this process from the beginning, including creating a link_token
, sending the customer through the Link flow, generating a new bank account token, and creating a new customer object or Custom account.
1// Change sandbox to production when you're ready to go live!2const {3 Configuration,4 PlaidApi,5 PlaidEnvironments,6 ProcessorStripeBankAccountTokenCreateRequest,7} = require('plaid');8const configuration = new Configuration({9 basePath: PlaidEnvironments[process.env.PLAID_ENV],10 baseOptions: {11 headers: {12 'PLAID-CLIENT-ID': process.env.PLAID_CLIENT_ID,13 'PLAID-SECRET': process.env.PLAID_SECRET,14 'Plaid-Version': '2020-09-14',15 },16 },17});18
19const plaidClient = new PlaidApi(configuration);20
21try {22 // Exchange the public_token from Plaid Link for an access token.23 const tokenResponse = await plaidClient.itemPublicTokenExchange({24 public_token: publicToken,25 });26 const accessToken = tokenResponse.data.access_token;27
28 // Generate a bank account token29 const request: ProcessorStripeBankAccountTokenCreateRequest = {30 access_token: accessToken,31 account_id: accountID,32 };33 const stripeTokenResponse = await plaidClient.processorStripeBankAccountTokenCreate(34 request,35 );36 const bankAccountToken = stripeTokenResponse.data.stripe_bank_account_token;37} catch (error) {38 // handle error39}
For a valid request, the API will return a JSON response similar to:
1{2 "stripe_bank_account_token": "btok_5oEetfLzPklE1fwJZ7SG",3 "request_id": "[Unique request ID]"4}
For possible error codes, see the full listing of Plaid error codes.
Note: The account_id
parameter is required if you wish to receive a Stripe bank account token.
Test with Sandbox credentials
You can create Stripe bank account tokens in all three API environments:
- Sandbox (https://sandbox.plaid.com): test simulated users with Stripe's "test mode" API
- Production (https://production.plaid.com): production environment for when you're ready to go live
Plaid's Sandbox API environment is compatible with Stripe's "test mode" API. To test the integration in Sandbox mode, simply use the Plaid Sandbox credentials when launching Link with a link_token
created in the Sandbox environment. The Stripe bank account token created in the Sandbox environment will always match the Stripe bank test account with account number 000123456789 and routing number 110000000, and with the Stripe account that is linked in the Plaid Dashboard.
Use Stripe's ACH API in test mode to create test transfers using the bank account tokens you retrieve from Plaid's Sandbox API environment.
When testing in the Sandbox, you have the option to use the /sandbox/public_token/create
endpoint instead of the end-to-end Link flow to create a public_token
. When using the /sandbox/public_token/create
-based flow, the Account Select flow will be bypassed and the accounts
array will not be populated. On Sandbox, instead of using the accounts
array, you can call /accounts/get
and test with any returned account ID associated with an account with the subtype checking
or savings
.
Get ready for production
Your account is immediately enabled for our Sandbox environment (https://sandbox.plaid.com), which allows you to test with Sandbox API credentials. To move to Production, request access from the Dashboard.
Next steps
Once you're successfully retrieving Stripe bank_account_token
s, you're ready to make ACH transactions with Stripe. Head to Stripe's ACH guide to get started, and if you have any issues, please reach out to Stripe Support.
If you are using the Charges and Sources API, the Stripe documentation will walk you through the process.
If you are using the Payment Intents API, you will first need to create a Stripe customer using the bank account token, then follow the migration guide to create a Payment Intent based on the bank account, using the manual migration flow. Note that you will need to contact Stripe to request access to this flow.
Example code in Plaid Pattern
For a real-life example of an app that integrates a processor partner, see the Node-based Plaid Pattern Account Funding sample app. Pattern Account Funding is a sample account funding app that creates a processor token to send to your payment partner. (Note that while the processor token creation call in Plaid Pattern's items.js demonstrates usage of the /processor/token/create
endpoint for creating a processor token, Stripe integrations should use the /processor/stripe/bank_account_token/create
endpoint instead and create a bank account token.)
Troubleshooting
Stripe bank account token not returned
When a stripe_bank_account_token
is not returned, a typical cause is that your Stripe and Plaid accounts have not yet been linked. First, be sure that your Stripe account has been configured to accept ACH transfers. Then, link your Stripe account to your Plaid account and re-try your request.
Stripe::InvalidRequestError: No such token
The Stripe::InvalidRequestError: No such token
error is returned by Stripe when the bank_account_token
could not be used with the Stripe API. Make sure you are not mixing Plaid's Sandbox environment with Stripe's production environment and that, if you have more than one Stripe account or more than one Plaid client_id
, you are using the Stripe account that is linked to the Plaid client_id
you are using.
Support and questions
Find answers to many common integration questions and concerns, such as pricing, sandbox and test mode usage in our docs.
If you're still stuck, open a support ticket with information describing the issue that you're experiencing and we'll get back to you as soon as we can.