Get started with the Quickstart
A quick introduction to building with Plaid
Want more video tutorials? The full getting started guide for the Quickstart app is available on YouTube.
Don't want to write code? Check out the Plaid Postman Collection for a no-code way to get started with Plaid's API.
Looking to get started with Identity Verification or Income Verification? For Identity Verification, check out the Identity Verification Quickstart. For Income, try the Income Verification Starter app.
Introduction
Let’s test out running Plaid locally by cloning the Quickstart app. You’ll need API keys, which you can receive by signing up in the Dashboard.
You'll have two different API keys, and there are three different Plaid environments. Today we'll start in the Sandbox environment. View the API Keys section of the Dashboard to find your Sandbox secret.
API Key
View Keys in DashboardEnvironment
If you get stuck at any point in the Quickstart, help is just a click away! Check the Quickstart troubleshooting guide or ask other developers in our Stack Overflow community.
Quickstart setup
Once you have your API keys, it's time to run the Plaid Quickstart locally! The instructions below will guide you through the process of cloning the Quickstart repository, customizing the .env file with your own Plaid client ID and Sandbox secret, and finally, building and running the app.
Plaid offers both Docker and non-Docker options for the Quickstart. If you don't have Docker installed, you may wish to use the non-Docker version; this path is especially recommended for Windows users who do not have Docker installations. However, if you already have Docker installed, we recommend the Docker option because it is simpler and easier to run the Quickstart. Below are instructions on setting up the Quickstart with Docker and non-Docker configurations.
Select group for content switcherSetting up without Docker
Make sure you have npm installed before following along. If you're using Windows, ensure you have a terminal capable of running basic Unix shell commands.
1# Note: If on Windows, run2# git clone -c core.symlinks=true https://github.com/plaid/quickstart3# instead to ensure correct symlink behavior4
5git clone https://github.com/plaid/quickstart.git6
7# Copy the .env.example file to .env, then fill8# out PLAID_CLIENT_ID and PLAID_SECRET in .env9cp .env.example .env10
11cd quickstart/node12
13# Install dependencies14npm install15
16# Start the backend app17./start.sh
Open a new shell and start the frontend app. Your app will be running at http://localhost:3000
.
1# Install dependencies2cd quickstart/frontend3npm install4
5# Start the frontend app6npm start7
8# Go to http://localhost:3000
Visit localhost and log in with Sandbox credentials (typically user_good
and
pass_good
, as indicated at the bottom of the page).
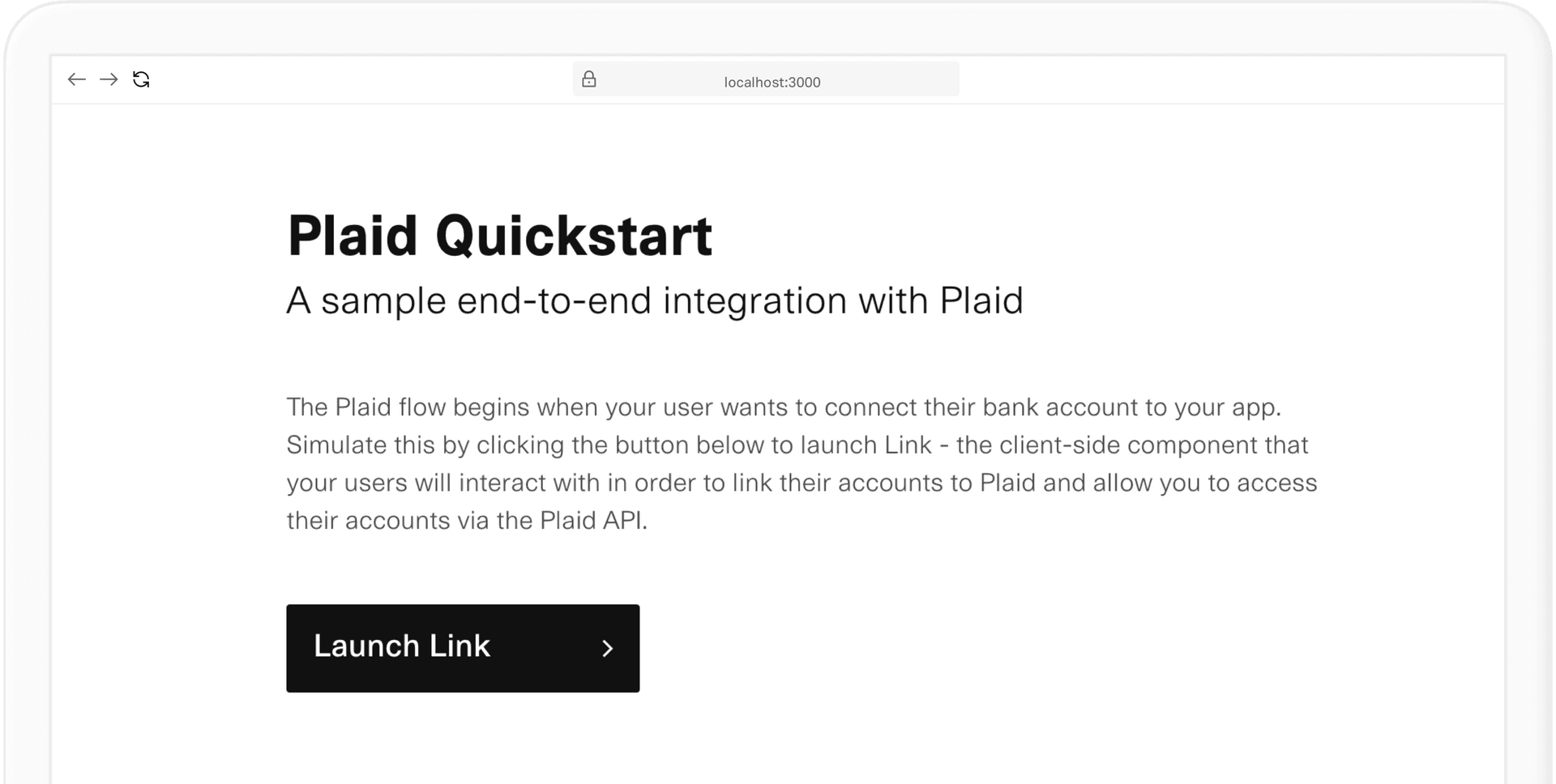
Setting up with Docker
Docker is a software platform that packages software into standardized units called containers that have everything the software needs to run, including libraries, system tools, code, and runtime. If you don't already have Docker, you can download it from the Docker site. Note that Windows users may need to take some additional steps, such installing a Linux environment; if you are using Windows and do not already have a Linux environment installed, we recommend selecting the non-Docker instructions instead.
Once Docker is installed, launch the Docker app, then use the following commands at the command line to configure and run the Quickstart. If the make
commands do not work, ensure that Docker is running. You may also need to prefix the make
commands with sudo
, depending on your environment.
1# Note: If on Windows, run2# git clone -c core.symlinks=true https://github.com/plaid/quickstart3# instead to ensure correct symlink behavior4
5git clone https://github.com/plaid/quickstart.git6cd quickstart7
8# Copy the .env.example file to .env, then fill9# out PLAID_CLIENT_ID and PLAID_SECRET in .env10cp .env.example .env11
12# start the container for one of these languages:13# node, python, java, ruby, or go14
15make up language=node16
17# Go to http://localhost:3000
Visit localhost and log in with Sandbox credentials (typically user_good
and
pass_good
, as indicated at the bottom of the page).
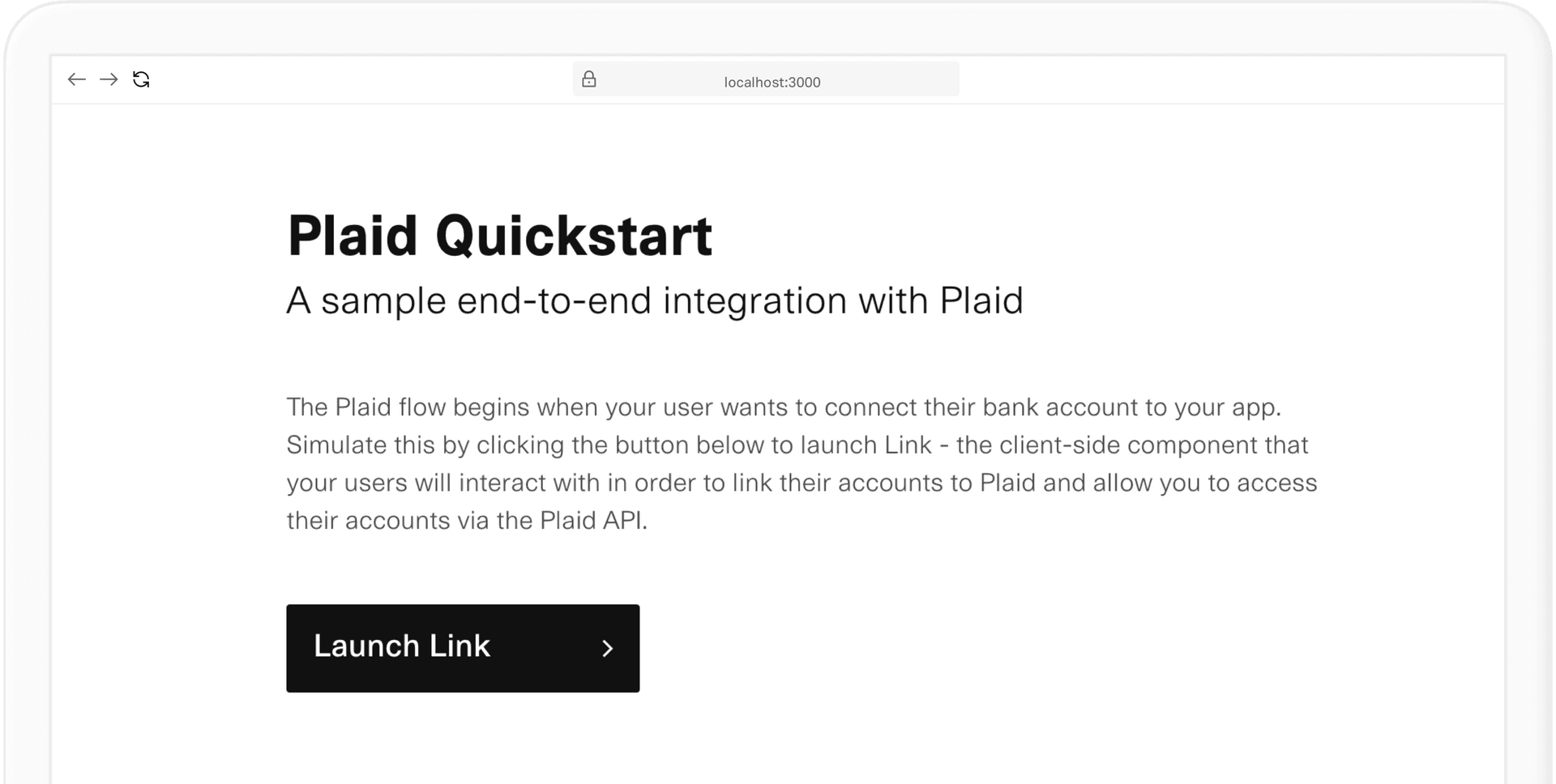
1$ make logs language=node
1$ make stop language=node
Create your first Item
Most API requests interact with an Item, which is a Plaid term for a login at a financial institution. A single end-user of your application might have accounts at different financial institutions, which means they would have multiple different Items. An Item is not the same as a financial institution account, although every account will be associated with an Item. For example, if a user has one login at their bank that allows them to access both their checking account and their savings account, a single Item would be associated with both of those accounts.
Now that you have the Quickstart running, you’ll add your first Item in the Sandbox environment. Once you’ve opened the Quickstart app on localhost, click the Launch Link button and select any institution. Use the Sandbox credentials to simulate a successful login.
Sandbox credentials
1username: user_good2password: pass_good3If prompted to enter a 2FA code: 1234
Once you have entered your credentials and moved to the next screen, you have created your first Item! You can now make API calls for that Item by using the buttons in the Quickstart. In the next section, we'll explain what actually happened and how the Quickstart works.
How it works
As you might have noticed, you use both a server and a client-side component to access the Plaid APIs. The flow looks like this:
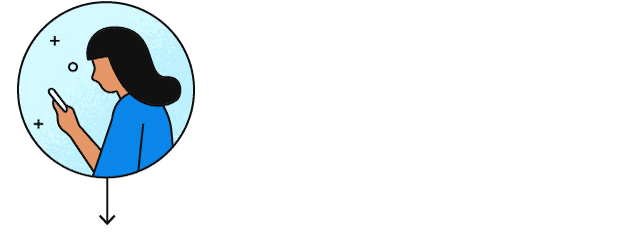
/link/token/create
to create a link_token
and pass the temporary token to your app's client.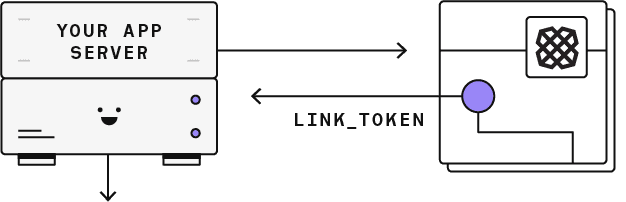
link_token
to open Link for your user. In the onSuccess
callback, Link will provide a temporary public_token
. This token can also be obtained on the backend via `/link/token/get`.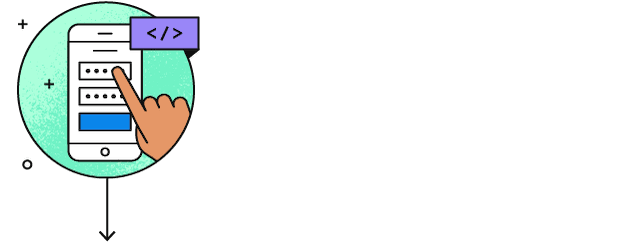
/item/public_token/exchange
to exchange the public_token
for a permanent access_token
and item_id
for the new Item
.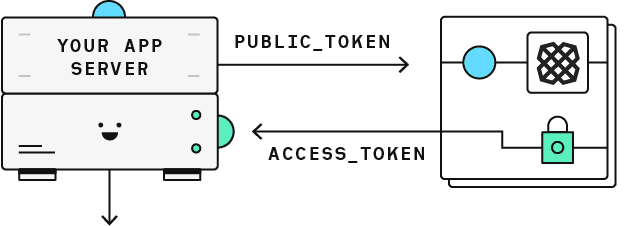
access_token
and use it to make product requests for your user's Item
.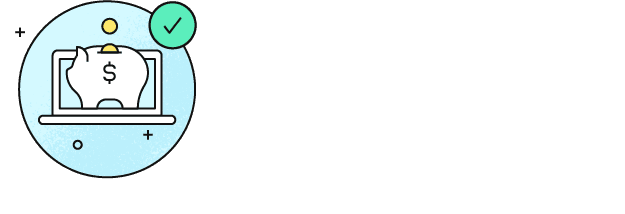
The first step is to create a new link_token
by making a /link/token/create
request and passing in the required configurations. This link_token
is a short lived, one-time use token that authenticates your app with Plaid Link, our frontend module. Several of the environment variables you configured when launching the Quickstart, such as PLAID_PRODUCTS
, are used as parameters for the link_token
.
1app.post('/api/create_link_token', async function (request, response) {2 // Get the client_user_id by searching for the current user3 const user = await User.find(...);4 const clientUserId = user.id;5 const request = {6 user: {7 // This should correspond to a unique id for the current user.8 client_user_id: clientUserId,9 },10 client_name: 'Plaid Test App',11 products: ['auth'],12 language: 'en',13 webhook: 'https://webhook.example.com',14 redirect_uri: 'https://domainname.com/oauth-page.html',15 country_codes: ['US'],16 };17 try {18 const createTokenResponse = await client.linkTokenCreate(request);19 response.json(createTokenResponse.data);20 } catch (error) {21 // handle error22 }23});
Once you have a link_token
, you can use it to initialize Link. Link is a drop-in client-side module available for web, iOS, and Android that handles the authentication process. The Quickstart uses Plaid's optional React bindings for an integration that you trigger via your own client-side code. This is what your users use to log into their financial institution accounts.
After a user submits their credentials within Link, Link provides you with a public_token
via the onSuccess
callback. The code below shows how the Quickstart passes the public_token
from client-side code to the server. Both React and vanilla JavaScript examples are shown.
1// APP COMPONENT2// Upon rendering of App component, make a request to create and3// obtain a link token to be used in the Link component4import React, { useEffect, useState } from 'react';5import { usePlaidLink } from 'react-plaid-link';6const App = () => {7 const [linkToken, setLinkToken] = useState(null);8 const generateToken = async () => {9 const response = await fetch('/api/create_link_token', {10 method: 'POST',11 });12 const data = await response.json();13 setLinkToken(data.link_token);14 };15 useEffect(() => {16 generateToken();17 }, []);18 return linkToken != null ? <Link linkToken={linkToken} /> : <></>;19};20// LINK COMPONENT21// Use Plaid Link and pass link token and onSuccess function22// in configuration to initialize Plaid Link23interface LinkProps {24 linkToken: string | null;25}26const Link: React.FC<LinkProps> = (props: LinkProps) => {27 const onSuccess = React.useCallback((public_token, metadata) => {28 // send public_token to server29 const response = fetch('/api/set_access_token', {30 method: 'POST',31 headers: {32 'Content-Type': 'application/json',33 },34 body: JSON.stringify({ public_token }),35 });36 // Handle response ...37 }, []);38 const config: Parameters<typeof usePlaidLink>[0] = {39 token: props.linkToken!,40 onSuccess,41 };42 const { open, ready } = usePlaidLink(config);43 return (44 <button onClick={() => open()} disabled={!ready}>45 Link account46 </button>47 );48};49export default App;
Next, on the server side, the Quickstart calls /item/public_token/exchange
to obtain an access_token
, as illustrated in the code excerpt below. The access_token
uniquely identifies an Item and is a required argument for most Plaid API endpoints. In your own code, you'll need to securely store your access_token
in order to make API requests for that Item.
1app.post('/api/exchange_public_token', async function (2 request,3 response,4 next,5) {6 const publicToken = request.body.public_token;7 try {8 const response = await client.itemPublicTokenExchange({9 public_token: publicToken,10 });11
12 // These values should be saved to a persistent database and13 // associated with the currently signed-in user14 const accessToken = response.data.access_token;15 const itemID = response.data.item_id;16
17 res.json({ public_token_exchange: 'complete' });18 } catch (error) {19 // handle error20 }21});
Making API requests
Now that we've gone over the Link flow and token exchange process, we can explore what happens when you press a button in the Quickstart to make an API call. As an example, we'll look at the Quickstart's call to /accounts/get
, which retrieves basic information, such as name and balance, about the accounts associated with an Item. The call is fairly straightforward and uses the access_token
as a single argument to the Plaid client object.
1app.get('/api/accounts', async function (request, response, next) {2 try {3 const accountsResponse = await client.accountsGet({4 access_token: accessToken,5 });6 prettyPrintResponse(accountsResponse);7 response.json(accountsResponse.data);8 } catch (error) {9 prettyPrintResponse(error);10 return response.json(formatError(error.response));11 }12});
Example response data:
1{2 "accounts": [3 {4 "account_id": "A3wenK5EQRfKlnxlBbVXtPw9gyazDWu1EdaZD",5 "balances": {6 "available": 100,7 "current": 110,8 "iso_currency_code": "USD",9 "limit": null,10 "unofficial_currency_code": null11 },12 "mask": "0000",13 "name": "Plaid Checking",14 "official_name": "Plaid Gold Standard 0% Interest Checking",15 "subtype": "checking",16 "type": "depository"17 },18 {19 "account_id": "GPnpQdbD35uKdxndAwmbt6aRXryj4AC1yQqmd",20 "balances": {21 "available": 200,22 "current": 210,23 "iso_currency_code": "USD",24 "limit": null,25 "unofficial_currency_code": null26 },27 "mask": "1111",28 "name": "Plaid Saving",29 "official_name": "Plaid Silver Standard 0.1% Interest Saving",30 "subtype": "savings",31 "type": "depository"32 },33 {34 "account_id": "nVRK5AmnpzFGv6LvpEoRivjk9p7N16F6wnZrX",35 "balances": {36 "available": null,37 "current": 1000,38 "iso_currency_code": "USD",39 "limit": null,40 "unofficial_currency_code": null41 },42 "mask": "2222",43 "name": "Plaid CD",44 "official_name": "Plaid Bronze Standard 0.2% Interest CD",45 "subtype": "cd",46 "type": "depository"47 }48 ...49 ],50 "item": {51 "available_products": [52 "assets",53 "balance",54 "identity",55 "investments",56 "transactions"57 ],58 "billed_products": ["auth"],59 "consent_expiration_time": null,60 "error": null,61 "institution_id": "ins_12",62 "item_id": "gVM8b7wWA5FEVkjVom3ri7oRXGG4mPIgNNrBy",63 "webhook": "https://requestb.in"64 },65 "request_id": "C3IZlexgvNTSukt"66}
Next steps
Congratulations, you have completed the Plaid Quickstart! From here, we invite you to modify the Quickstart code in order to get more practice with the Plaid API. There are a few directions you can go in now:
Go to the docs homepage for links to product-specific documentation.
For more sample apps, including a bare-bones minimal Plaid Quickstart implementation and apps demonstrating real world examples of funds transfer and personal financial management, see sample apps.
Our YouTube playlist Plaid in 3 minutes has brief introductions to many Plaid products. For more detailed tutorial videos, see Plaid Academy.
Looking to move money with a Plaid partner, such as Dwolla? See Move money with our partners for partner-specific money movement Quickstarts.
The Quickstart covers working with web apps. If your Plaid app will be on mobile, see Plaid Link to learn about getting started with mobile client-side setup.